class: middle, center # Lecture 4 ### 31 August 2020 Unit 8: **Conditionals**
Unit 9: **Logical Expression**
Unit 10: **Assertion**
--- class: middle ## Tutorial/Lab 2 - Problem sets from Units 3, 5, and 8. - Prepare you for Assignment 1 --- class: middle ## Assignment 1 - Release this Thursday morning - Due next Tuesday 23:59 --- class: middle ## Survey - Are you coping with online lecture/lab in CS1010? - We want to know: Luminus -> CS1010 -> Survey --- class: middle ## Mock Exam Session - Register by 5pm TODAY - Read [SoC's Procedure for Mock Exam](https://mysoc.nus.edu.sg/wp-login.php?redirect_to=%2Facademic%2Fmock-e-exams-for-students%2F) --- class: middle ## Please read - [How Not to Go About a Programming Assignment](http://people.irisa.fr/Martin.Quinson/Teaching/how-not-to-code.pdf) - [About Assignments in CS1010](assignments.md) - [General CS1010 Policies](policy.md) --- class: middle
Problem Solving
decomposition
recursion
flowchart
C Language/Syntax
types
functions
arithmetic ops
Behavioural/Mental Model
machine code
data in memory
types
Tools/Good Practices
clang
vim
bash
--- class: middle
Problem Solving
decomposition
recursion
flowchart
conditionals
assertion
C Language/Syntax
types
functions
arithmetic ops
if-else
logical expression
Behavioural/Mental Model
machine code
data in memory
types
Tools/Good Practices
clang
vim
bash
--- class: middle,wide .fit[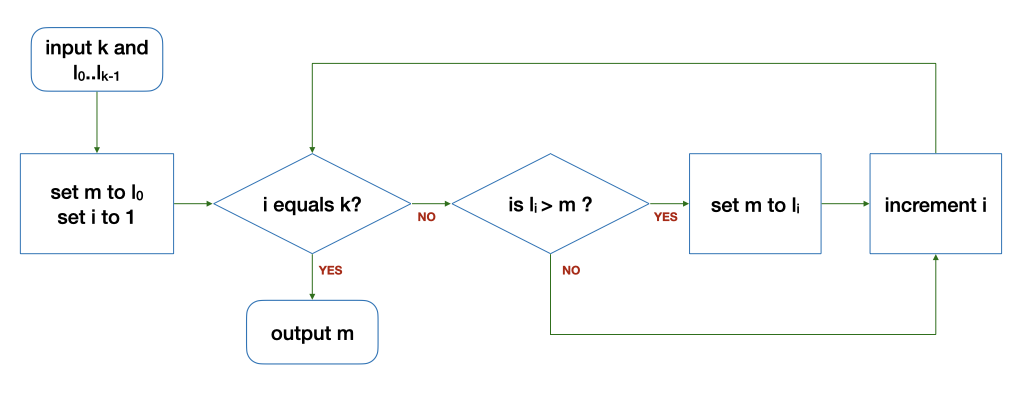] --- class: middle,wide .fit[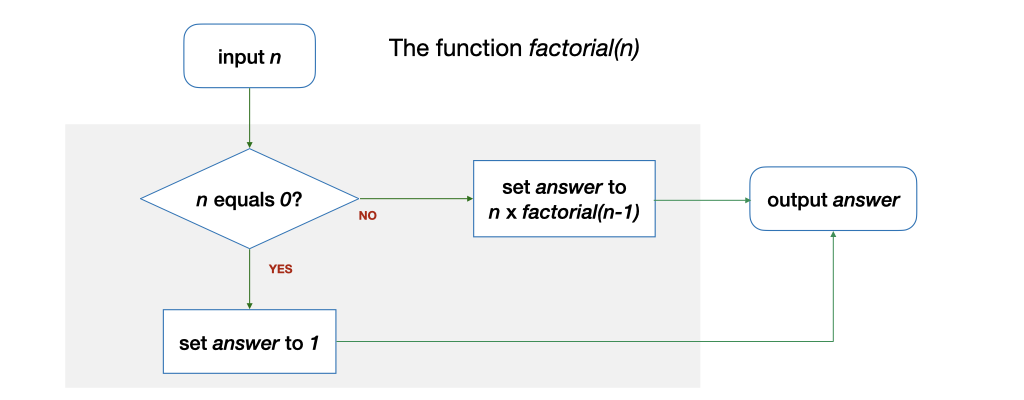] --- class: middle,wide .small[ ```C long factorial(long n) { long answer; if (n == 0) { answer = 1; } else { answer = n * factorial(n - 1); } return answer; } ``` ] --- class: middle | Score | Letter Grade | | --------------------------- | ------------ | | 8 or higher | A | | Less than 8 but 5 or higher | B | | Less than 5 but 3 or higher | C | | Less than 3 | D | --- class: middle | Score | Letter Grade | | ----------- | ------------ | | 8 or higher | A | | Less than 8 | See Table 1 | --- class: middle Table 1 (less than 8) | Score | Letter Grade | | ----------- | ------------ | | 5 or higher | B | | Less than 5 | See Table 2 | --- class: middle Table 2 (less than 5) | Score | Letter Grade | | ----------- | ------------ | | 3 or higher | C | | Less than 3 | D | --- class: middle .tiny[ ```C void print_score(double score) { if (score >= 8) { cs1010_println_string("A"); } else { // Table 1 if (score >= 5) { cs1010_println_string("B"); } else { // Table 2 if (score >= 3) { cs1010_println_string("C"); } else { cs1010_println_string("D"); } } } } ``` ] --- class: middle Rewritten with `else if` .tiny[ ```C void print_score(double score) { if (score >= 8) { cs1010_println_string("A"); } else if (score >= 5) { cs1010_println_string("B"); } else if (score >= 3) { cs1010_println_string("C"); } else { cs1010_println_string("D"); } } ``` ] --- class: middle This is accepted in C (but not CS1010) .tiny[ ```C void print_score(double score) { if (score >= 8) cs1010_println_string("A"); else if (score >= 5) cs1010_println_string("B"); else if (score >= 3) cs1010_println_string("C"); else cs1010_println_string("D"); } ``` ] --- class: middle When will cause `good effort` to be printed? .tiny[ ```C void print_score(double score) { if (score >= 8) cs1010_println_string("A"); else if (score >= 5) cs1010_println_string("B"); else if (score >= 3) cs1010_println_string("C"); else cs1010_println_string("D"); cs1010_println_string("good effort"); } ``` ] --- class: middle What will get printed in `score` is 5? .tiny[ ```C if (score >= 8) if (late_penalty != 0) cs1010_println_string("late submission"); else cs1010_println_string("you can do better!"); ``` ] --- class: middle,center .fit[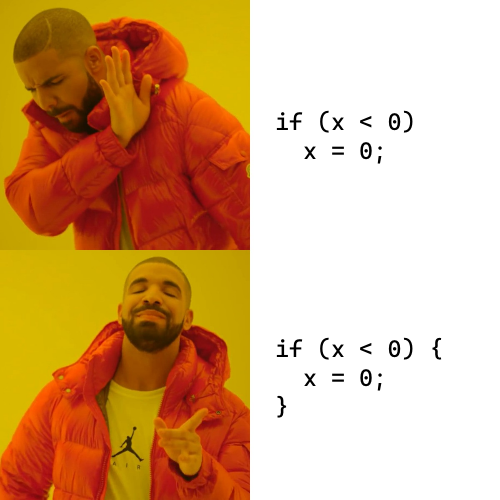] --- class: middle .smaller[ ```C if (max_so_far < x) { max_so_far = x; } else { } ``` can be simplified to ```C if (max_so_far < x) { max_so_far = x; } ``` ] --- class: middle Set `max` to the larger of `x` and `y` .smaller[ ```C if (x > y) { max = x; } if (x < y) { max = y; } ``` ] What is wrong? --- class: middle .smaller[ ```C if (x > y) { max = x; } if (x < y) { max = y; } if (x == y) { max = y; } ``` ] --- class: middle .smaller[ ```C if (x > y) { max = x; } if (x <= y) { max = y; } ``` ] --- class: middle .smaller[ ```C if (x > y) { max = x; } else { max = y; } ``` ] --- class: middle .smaller[ ```C if (x > y) { max = x; } else { max = y; } ``` ] --- class: middle ```C long factorial(long n) { long answer; if (n == 0) { answer = 1; } else { answer = n * factorial(n - 1); } return answer; } ``` ] --- class: middle ```C long factorial(long n) { if (n == 0) { return 1; } else { return n * factorial(n - 1); } } ``` --- class: middle `else` can be skipped ```C long factorial(long n) { if (n == 0) { return 1; } return n * factorial(n - 1); } ``` --- class: middle The `?:` operator. .tiny[ ```C long factorial(long n) { return n == 0 ? 1 : n * factorial(n - 1); } ``` ] --- class: middle,center .fit[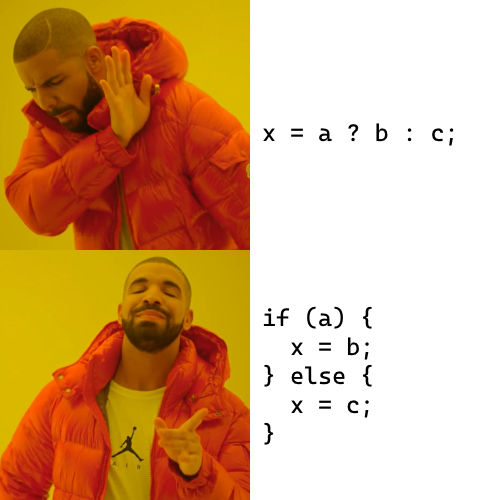] --- class: middle What could go wrong? .tiny[ ```C double z; if (z == 0.03) { : } ``` ] --- class: middle Comparing floating-point numbers: .tiny[ ```C double z; double e = 0.0000001; // epsilon if (fabs(z - 0.03) < e) { : } ``` ] --- class: middle,center .fit[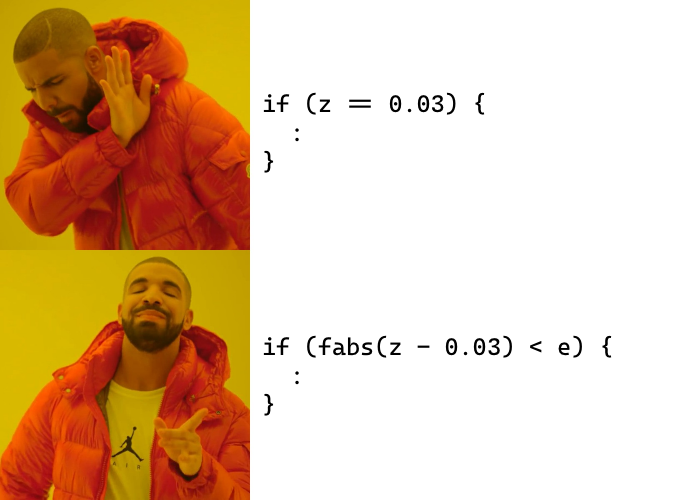] --- class: middle The `bool` type in C can take either the value `true` or `false`. To use: ```C #include
``` --- class: middle Old fashion C uses `int` as boolean. `0` for false, non-zero for true. ```C if (x - y) { } ``` --- class: middle,center .fit[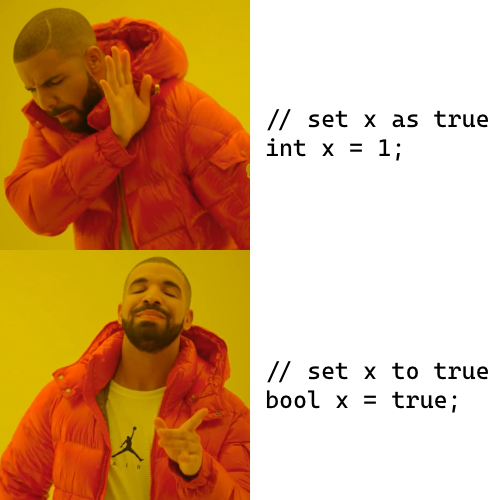] --- class: middle - Comparison operations : `>`, `<`, `>=`, `<=`, `==`, `!=` - Logical operators : `&&`, `||`, `!` --- class: middle,center ## De Morgan's Law `!(e1 && e2)` is the same as `(!e1) || (!e2)` --- class: middle,center ## De Morgan's Law `!(e1 || e2)` is the same as `(!e1) && (!e2)` --- class: middle,center ## Short Circuiting ### `a && b` ### `a || b` --- class: middle Which one is better? .tiny[ ```C if (number < 100000 && is_prime(number)) { : } ``` ] .tiny[ ```C if (is_prime(number) && number < 100000) { : } ``` ] --- class: middle,center ## Assertion ``` // { ... } ``` A logical expression that must be true at a given point in a program. --- class: middle ```C x = 1; // { x == 1 } ``` --- class: middle ```C if (x > y) { max = x; } else { max = y; } ``` how can we be sure that `max` is now the larger of the two? --- class: middle ```C if (x > y) { max = x; } else { max = y; } ``` --- class: middle .tiny[ ```C if (score >= 8) { cs1010_println_string("A"); } else { if (score >= 5) { cs1010_println_string("B"); } else { if (score >= 3) { cs1010_println_string("C"); } else { cs1010_println_string("D"); } } } ``` ] When will `C` gets printed? --- class: middle .tiny[ ```C if (score >= 8) { cs1010_println_string("A"); } else { if (score >= 5) { cs1010_println_string("B"); } else { if (score >= 3) { cs1010_println_string("C"); } else { cs1010_println_string("D"); } } } ``` ] When will `C` gets printed? --- class: bottom .tiny[ Version: 1.1 Last Updated: Mon Aug 31 11:05:14 +08 2020 ]