class: middle, center # Lecture 2 ### 16 August 2021 Admin Matters
**Unit 3**: Functions
**Unit 4**: Types --- class: middle,wide ### Reminders - Keep your mic muted unless you need my attention - Use Zoom Chat to discuss/chat among yourselves (I won't pay attention to it) - Use Pizza Live Q&A to ask me question during class --- class: middle,wide ### Reminders - My office hour: Tues 4-5pm, F2F and Online - Questions? Issues? Please use Piazza --- class: middle,wide ### Accounts - By now you should have your Piazza, GitHub, and SoC accounts setup and ready to go. - Target this week: - able to `ssh` into on PE environments - go through Unix CLI and `vim` tutorials --- class: middle,center ## Why Unix CLI? --- class: middle,center ## Why `vim`? --- class: middle,center .tiny[[via reddit](https://www.reddit.com/r/nus/comments/nwpc54/nus_cs_fun/h1b2ikz/?context=3)] .fit[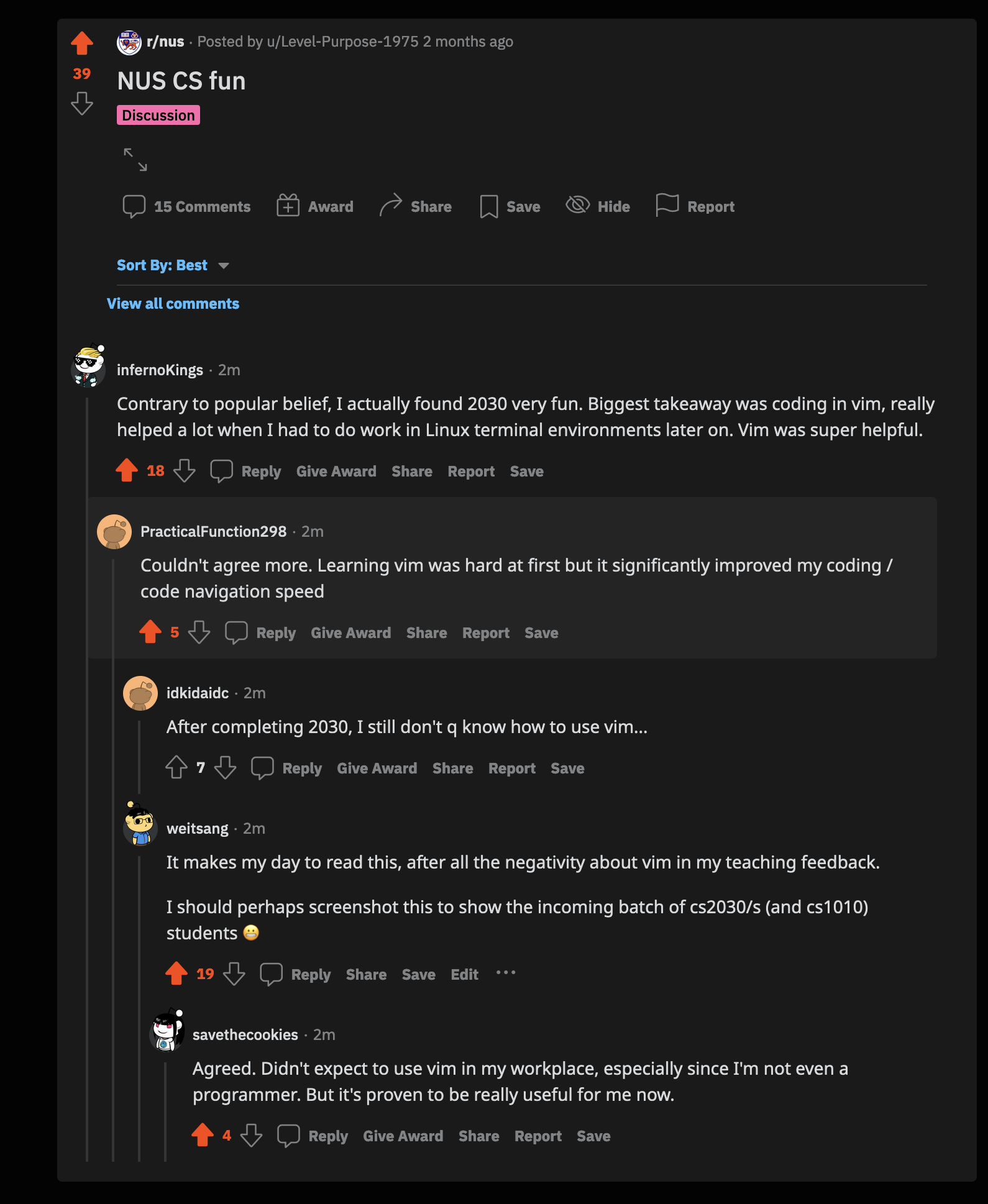] --- ### [Stackoverflow 2021 Developer Survey](https://insights.stackoverflow.com/survey/2021#section-most-loved-dreaded-and-wanted-collaboration-tools) .fit[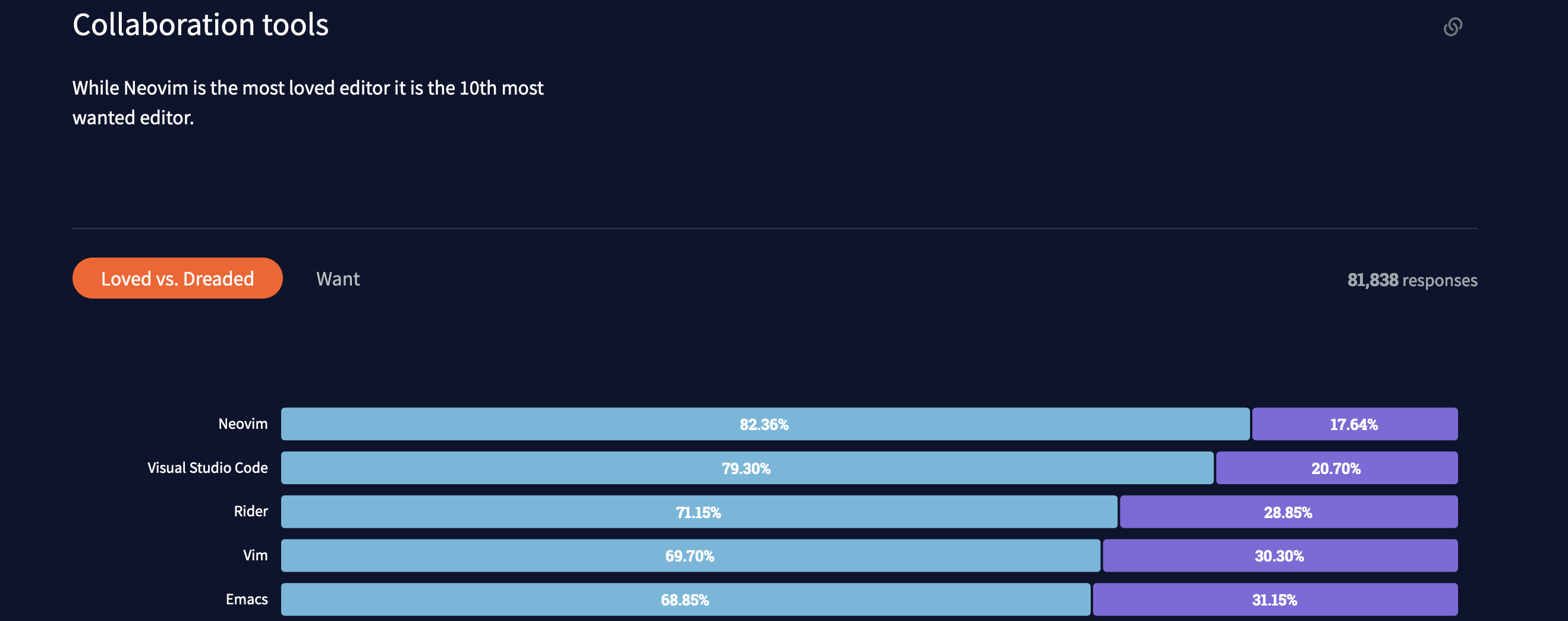] --- class: middle,wide ### Tutorials and Labs - Starts next week - Appeal via ModReg if you still haven't gotten a slot. - We might appeal to you to switch rooms to balanced out the groups (please say yes) --- class: middle,wide ### Tutorial 1 - Problem Set from Unit 2 ### Lab 1 - Setup, Unix, `vim`, Exercise 0 --- class: middle,wide .smaller[.fit[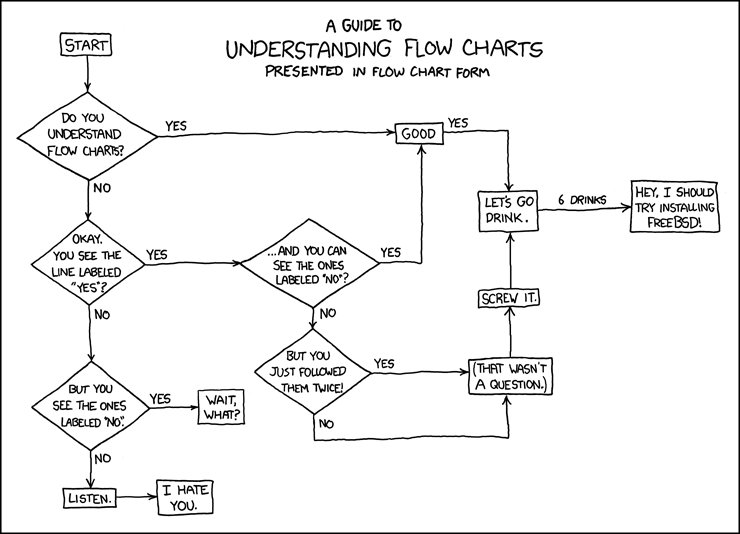]
https://xkcd.com/518] --- class: middle,wide ## Flowchart .fit[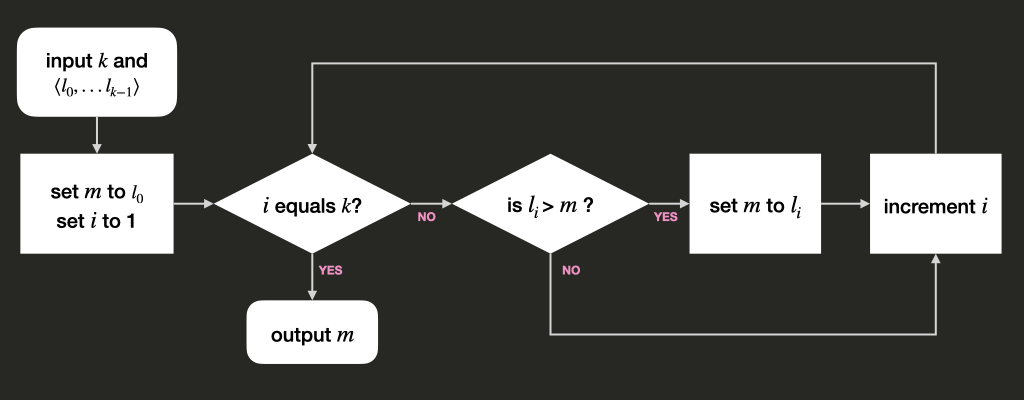] --- class: middle,center ## Functions --- class: middle,center,wide .fit[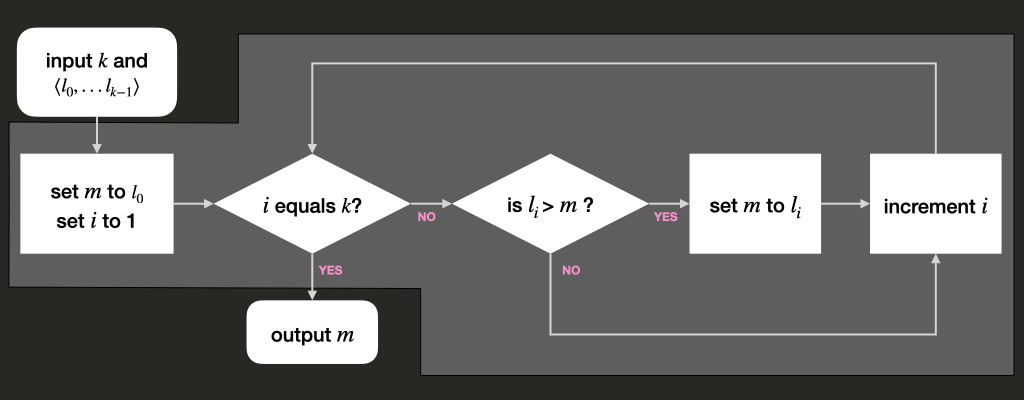] --- class: middle,center,wide .fit[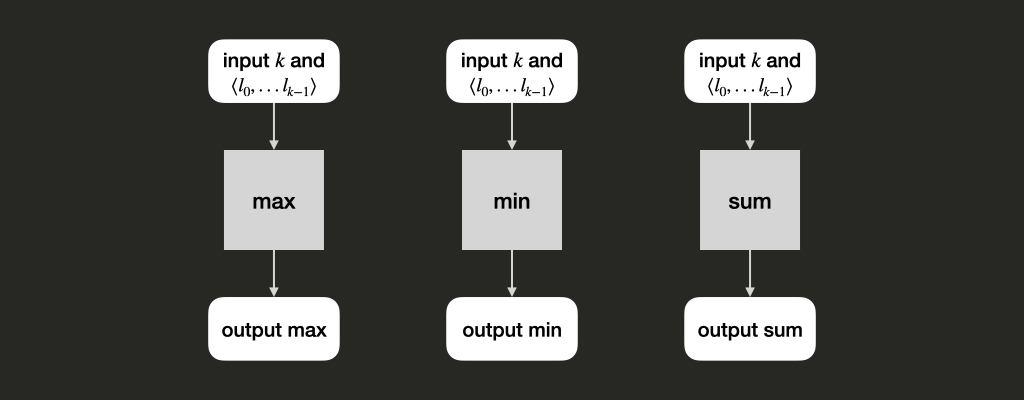] --- ### Find The Range - The _range_ of a given list of $k$ integers is the difference between the max and the min values in the list. - Give an algorithm to find the range given a list $L$ of $k$ integers. --- class: middle,center ## $max(L, k) - min(L, k)$ --- class: middle,center ### Refer to a previous solution to a sub-problem, which we assume we already know how to solve. --- class: middle,center ## Wishful Thinking --- class: middle ### Wishful Thinking - A powerful tool to help us think at a higher level. - We can focus on _what_ a function does, not _how_ it does it. --- class: middle,center ### A C program is just a collection of functions! --- class: middle ### A C program is just a collection of functions - written by you - provided by the standard C library - provided by other libraries --- class: middle ### Find the Mean - Give an algorithm to find the mean value given a list $L$ of $k$ integers. --- class: middle,center ## $\frac{sum(L,k)}{k}$ --- class: middle,center Assume $len(L)$ returns the size of the list. ## $\frac{sum(L)}{len(L)}$ --- class:middle ### Find the Std Dev - Give an algorithm to find the standard deviation of a list $L$ of $k$ integers. $$\sqrt{\frac{\sum\_{i=0}^{k-1}(l\_i - \mu)^2}{k}}$$ --- class:middle ## Let's break it down into sub-problems --- class: middle,center $$\sqrt{\frac{\sum\_{i=0}^{k-1}(l\_i - \mu)^2}{k}}$$ --- class: middle,center $$\sum\_{i=0}^{k-1}(l\_i - \mu)^2$$ set $\mu$ to $mean(L)$ set $L'$ to $subtract(L, \mu)$ set $L''$ to $square(L')$ set $total$ to $sum(L'')$ --- class: middle,center $$\sum\_{i=0}^{k-1}(l\_i - \mu)^2$$ $sum(square(subtract(L, mean(L))))$ --- class: middle,center $$\frac{\sum\_{i=0}^{k-1}(l\_i - \mu)^2}{k}$$ $mean(square(subtract(L, mean(L))))$ --- class: middle,center $$\sqrt\frac{\sum\_{i=0}^{k-1}(l\_i - \mu)^2}{k}$$ $sqrt(mean(square(subtract(L,mean(L)))))$ --- class: middle,center,wide .fit[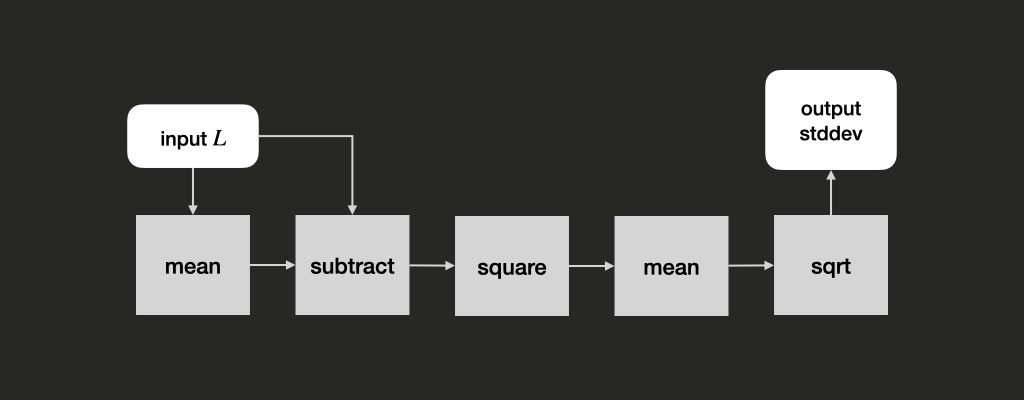] --- class:middle ### Wishful Thinking - $square(L)$ - $subtract(L, \mu)$ - $sqrt(x)$ --- class:middle,wide .fit[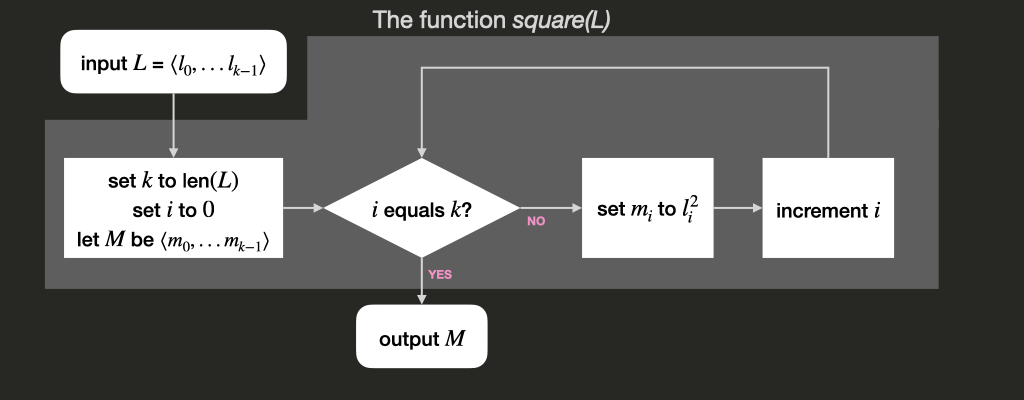] --- class:middle ### **IMPORTANT**: Break down a problem into sub-problems and solve them one-by-one with functions. Then compose the functions to solve the original given task. --- class: middle,center ### Find the maximum among these numbers ``` 5 9 8 1 3 2 ``` --- class: middle,center ### Find the maximum among these numbers .tiny[ ``` 12 20 11 20 14 2 24 36 43 16 27 6 11 7 10 15 38 10 22 7 16 26 32 30 11 9 30 6 20 6 27 19 26 10 27 6 19 34 5 9 3 22 10 4 13 1 10 22 43 36 39 29 41 12 13 25 17 8 30 31 29 38 2 42 7 45 24 33 9 40 34 29 37 2 26 17 19 34 6 7 34 22 21 41 38 5 15 13 9 1 42 39 5 29 38 4 22 29 41 10 26 32 30 26 16 16 18 22 32 34 14 10 5 17 25 16 19 6 31 16 3 13 8 42 41 0 13 30 44 1 41 14 5 39 40 38 6 37 38 9 . ``` ] --- class: middle,center ### Compare `12` to the maximum among .tiny[ ``` 20 11 20 14 2 24 36 43 16 27 6 11 7 10 15 38 10 22 7 16 26 32 30 11 9 30 6 20 6 27 19 26 10 27 6 19 34 5 9 3 22 10 4 13 1 10 22 43 36 39 29 41 12 13 25 17 8 30 31 29 38 2 42 7 45 24 33 9 40 34 29 37 2 26 17 19 34 6 7 34 22 21 41 38 5 15 13 9 1 42 39 5 29 38 4 22 29 41 10 26 32 30 26 16 16 18 22 32 34 14 10 5 17 25 16 19 6 31 16 3 13 8 42 41 0 13 30 44 1 41 14 5 39 40 38 6 37 38 9 . ``` ] --- class: middle,center ### Wishful Thinking We know how to find $max(L,k)$ for smaller $k$ --- class: middle ### Let $max'(L, i, j)$ returns the maximum among $l\_i, l\_{i+1} \ldots l\_j$ ### So $max(L, k)$ is just $max'(L, 0, k-1)$ --- class: middle ### if $i \not = j$, then $max'(L, i, j)$ is the larger between - $l\_i$ and - $max'(L, i+1, j)$ --- class: middle ### if $i$ equals $j$, $max'(L, i, j)$ is just $l_i$ --- --- class: middle,wide .fit[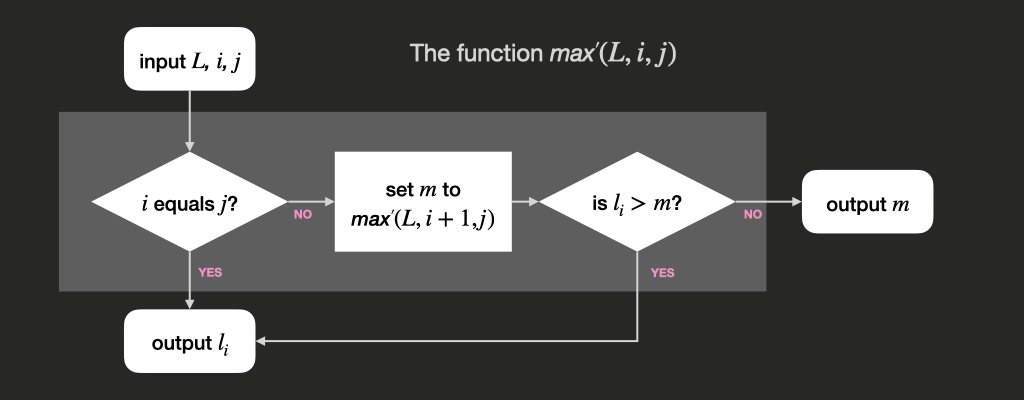] --- class: middle,center ### Find the maximum among .tiny[ ``` 12 20 11 20 14 2 24 36 43 16 27 6 11 7 10 15 38 10 22 7 16 26 32 30 11 9 30 6 20 6 27 19 26 10 27 6 19 34 5 9 3 22 10 4 13 1 10 22 43 36 39 29 41 12 13 25 17 8 30 31 29 38 2 42 7 45 24 33 9 40 34 29 37 2 26 17 19 34 6 7 34 22 21 41 38 5 15 13 9 1 42 39 5 29 38 4 22 29 41 10 26 32 30 26 16 16 18 22 32 34 14 10 5 17 25 16 19 6 31 16 3 13 8 42 41 0 13 30 44 1 41 14 5 39 40 38 6 37 38 9 . ``` ] --- class: middle,center ### Wishful Thinking We know how to find $max(L,k)$ for smaller $k$ --- class: middle,center ### Compare the maximum among these numbers .tiny[ ``` 12 20 11 20 14 2 24 10 15 38 10 22 7 16 20 6 27 19 26 10 27 10 4 13 1 10 22 43 17 8 30 31 29 38 2 34 29 37 2 26 17 19 38 5 15 13 9 1 42 41 10 26 32 30 26 16 5 17 25 16 19 6 31 13 30 44 1 41 14 5 . ``` ] --- class: middle,center ### With the maximum among these numbers .tiny[ ``` 36 43 16 27 6 11 7 26 32 30 11 9 30 6 6 19 34 5 9 3 22 36 39 29 41 12 13 25 42 7 45 24 33 9 40 34 6 7 34 22 21 41 39 5 29 38 4 22 29 16 18 22 32 34 14 10 16 3 13 8 42 41 0 39 40 38 6 37 38 9 . ``` ] --- class: middle ### if $j \not= i$, $max(L,i,j)$ is the larger between - $max'(L, i, n)$ and - $max'(L, n+1, j)$ where $n = \left\lfloor\frac{i+j}{2}\right\rfloor$ --- class: middle ### if $j$ equals $i$, $max(L,i,j)$ is just $l_i$. --- --- class: center,wide .fit[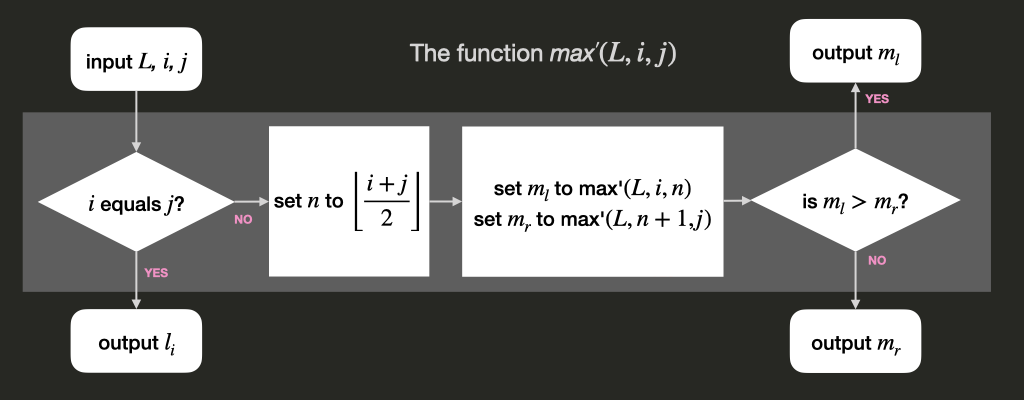] --- --- class: middle, center ## Divide and Conquer --- class: middle ## Find the factorial of $n$ - $n! = n \times n-1 \times \ldots \times 2 \times 1$ - $n! = n \times (n-1)!$ - Special case: $0! = 1$ --- class: middle .fit[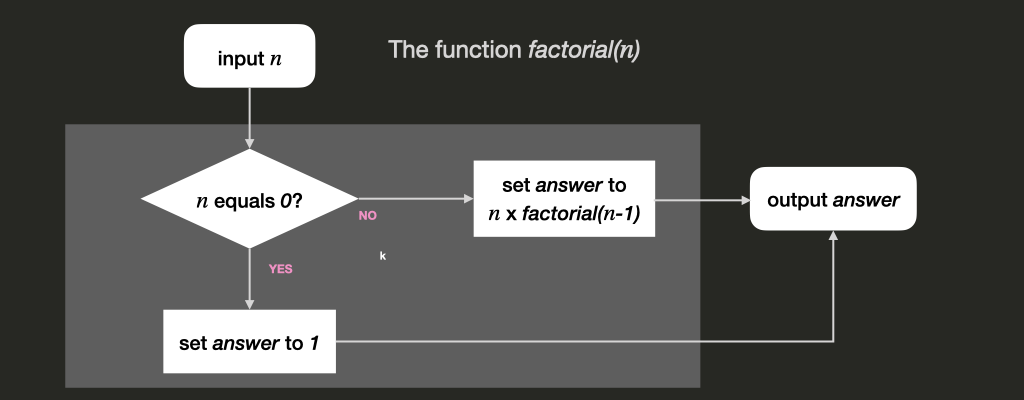] --- class:middle,center ## Recursion ### A function calling itself --- class:middle,center # Types --- class:middle ### `1010010101010101001111010..` can mean - 1933091 - 34.95108 - `hello` - an image --- class:middle ### A variable needs a type to be interpreted correctly. --- class:middle ### A type is represented by a fixed, finite, number of bits. ### Different types can be represented by a different number of bits. --- class:middle, center ### 1 bit: `1` or `0` black or white
true or false
yes or no
S or U --- class:middle, center ### 2 bits: `11`, `10`, `01`, or `00` north, south, east, west
spring, summer, fall, winter --- class:middle, center ### In general, $k$ bits can represent $2^k$ values --- class:middle ### more bits $\rightarrow$ more memory, but can represent bigger range ### fewer bits $\rightarrow$ less memory, but represent smaller range --- class:middle ### When writing programs for embedded systems, IoT devices, sensors, etc, you need to be frugal with memory usage. ### In CS1010, however, we will go with more bits for better precision. --- class:middle, center ## Integers --- class:middle, center ### 8 bits: 256 different integers 0 to 255 (for unsigned)
-128 to 127 (for signed) --- class:middle, center ### 64 bits signed -9,223,372,036,854,775,808
to
9,223,372,036,854,775,807 --- class:middle, center ## Characters --- class:middle, center ### 8 bits: 127 different symbols using ASCII standards ``` 0-9, A-Z, a-z <>?:”{}!@#$%^&* ()_+-=[]\';/., ``` return, tab, escape, etc --- class:middle, center ### up to 32 bits: Unicode (UTF-8) 11111011000000000 😀 --- class:middle, center ## Real numbers (also called floating point numbers) --- class:middle ### There are infinitely many real numbers but only a finite sequence of bits. ### Not all of real numbers can be represented. --- class:middle, center ### Not every real number can be presented precisely E.g., `0.1 + 0.2` is `0.30000000000000004` --- class:middle, center .fit[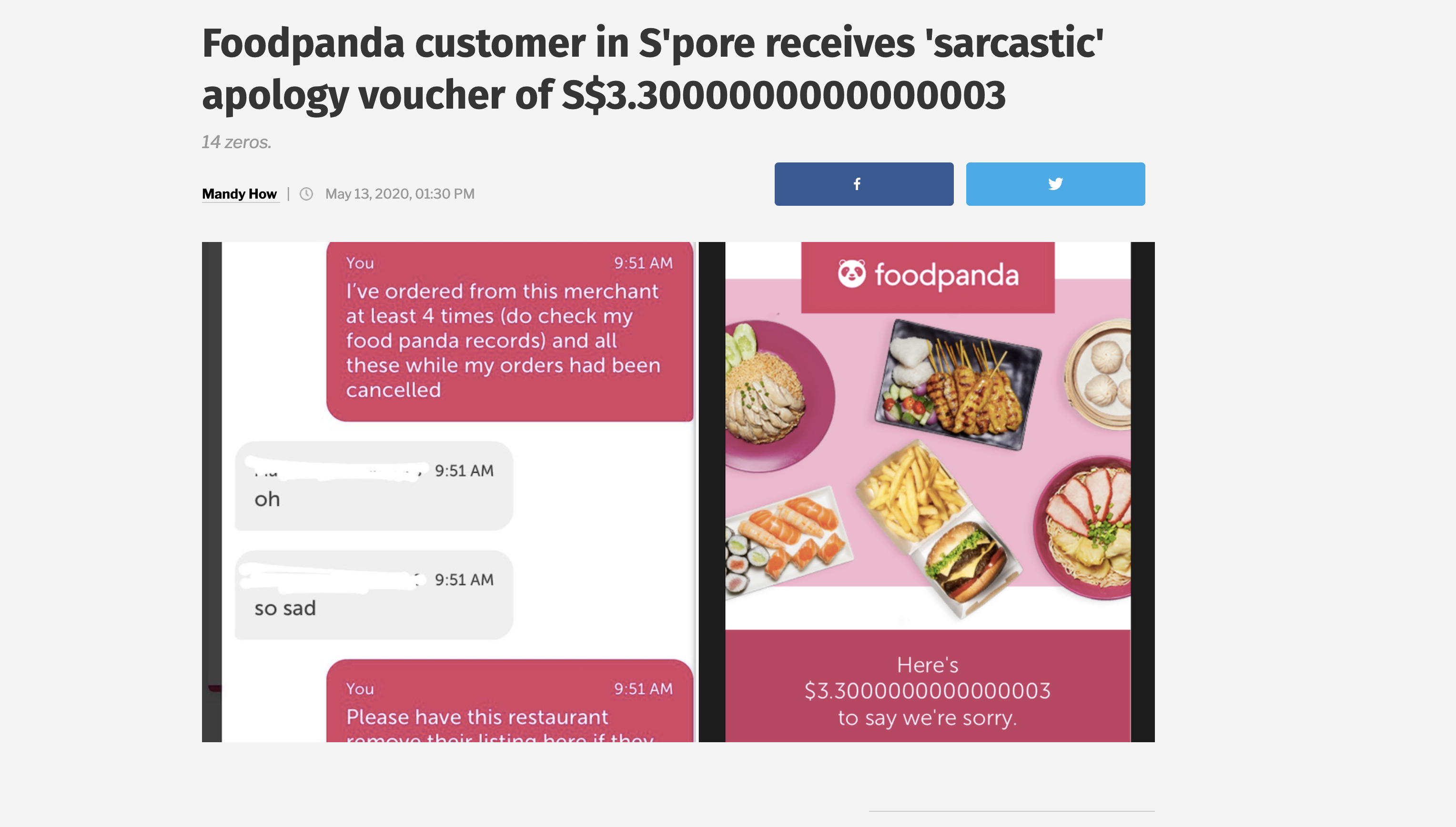] --- class:middle, center ### Rule: Use integer type unless you absolutely need to use a real number type. --- class:middle, center ### We normally use 32, 64, or 128 bits to represent real numbers. --- class:middle, center ### A variable needs a type to be interpreted correctly. --- class:middle, center set $total$ to $sum(L, k)$
set $avg$ to $mean(L, k)$ $k$ is an integer
$total$ is an integer
$avg$ must be a real number --- class:middle ## Homework - Problem Set in Unit 3 - `ssh` into CS1010 PE hosts and follow the Unix CLI and `vim` tutorial - Diagnostic Quiz for Lecture 2 (due this Wed) - Diagnostic Quiz for using CS1010 PE (due next Wed) --- class: bottom .tiny[ Version: 1.1 Last Updated: Tue Aug 18 12:24:32 +08 2020 ]