class: middle, center # Lecture 3 ### 26 August 2024 Admin Matters
Unit 5: **First C Program**
Unit 6: **CS1010 I/O Library**
Unit 7: **Arithmetic Operations**
--- ### Exercises - Read the [exercise guide](https://nus-cs1010.github.io/2425-s1/guides/exercises.html) - Read policy about: - [late submission](https://nus-cs1010.github.io/2425-s1/about.html#latemissed-submission-policy) - [collaboration](https://nus-cs1010.github.io/2425-s1/about.html#collaboration-on-programming-exercises) - [use of AI](https://nus-cs1010.github.io/2425-s1/about.html#use-of-ai-tools) - Read about [how to ask for help](https://nus-cs1010.github.io/2425-s1/about.html#asking-questions-and-getting-help) --- - Exercises are meant to help you learn. - Submitting a perfect solution is not the point. - Do not copy verbatim from AI, others, or the Internet. You will not learn anything. - Making mistakes is a critical part of the learning process. --- ### Collaboration - Help each other learn by _teaching each other_ the concepts and thought process behind. - Copy-pasting of code does _NOT_ help each other learn. - Give credits to those that help you. --- ### Catch-Up Session I - __Date__: Saturday, 31 August, 2024 - __Time__: 10 AM to 12 PM - Via [Zoom](https://nus-sg.zoom.us/j/7308691994?omn=86463549431) --- class: .small[ ### Catch-Up Session I - Optional session - Q&A format - Ask via [PigeonHole](https://pigeonhole.at/FUNCTIONSEVERYWHERE) (code: `FUNCTIONSEVERYWHERE`) - No new materials will be covered - Will be recorded ] --- .small[ To follow along today's lecture, copy the following three files from the home directory of `cs1010` to your working directory: - `Makefile` - `compile_flags.txt` - `.clang-tidy` **Note**: Every weekly programming exercise comes with three files with the same name. Don't mix them up. **Note**: My `vim` environment is already [set up according to here](https://nus-cs1010.github.io/2425-s1/guides/vim-setup.html). Yours might behave differently. ] --- ### Mathematical functions - Function declaration: $f : \mathbb{Z} \rightarrow \mathbb{Z}$ - Function definition: $f(x) = x^2$ - Referring to a function: $g(x) = f(x) + x$ - Evaluating a function: $f(4)$ evaluates to 16 --- class: middle,center .w20[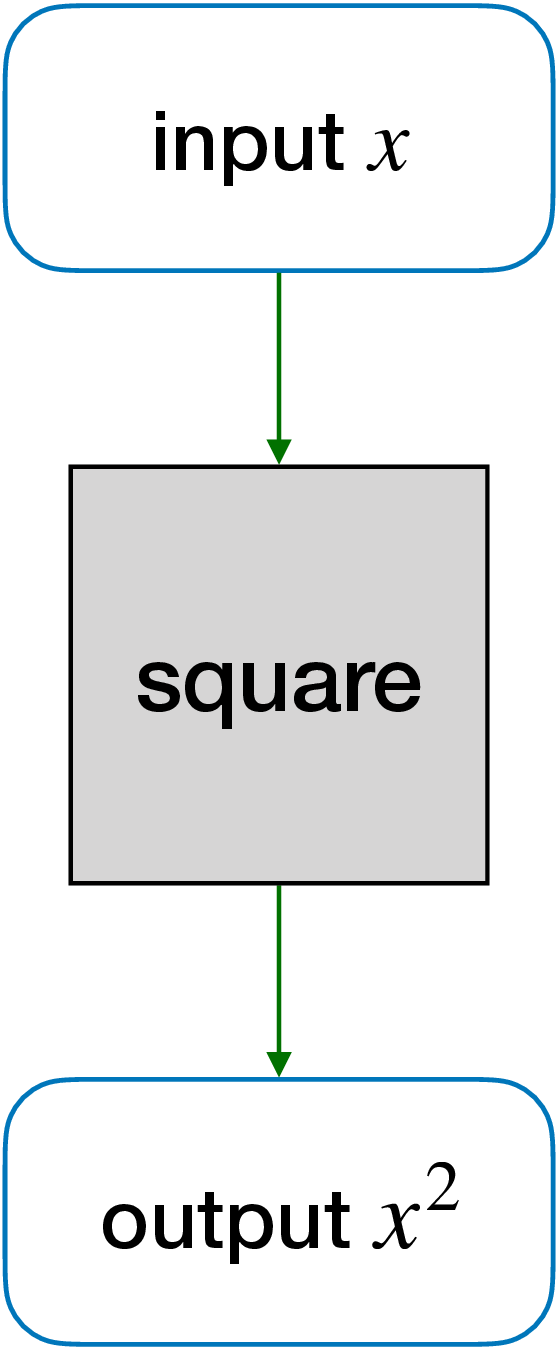] --- class: middle,center ## Let's code --- .fit[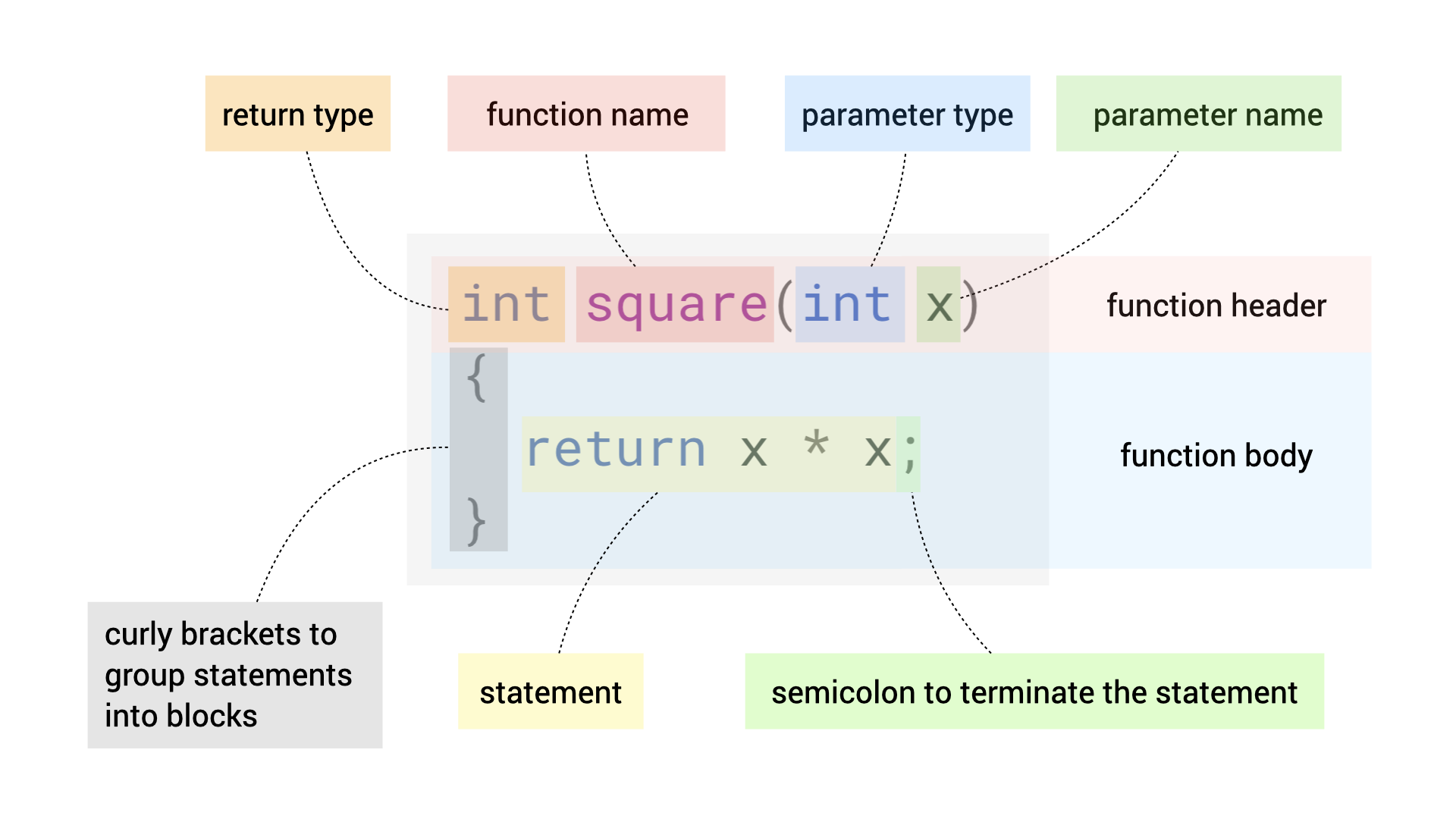] --- ### Compilation A compiler _compiles_ a program written in high-level language to machine code. .fit[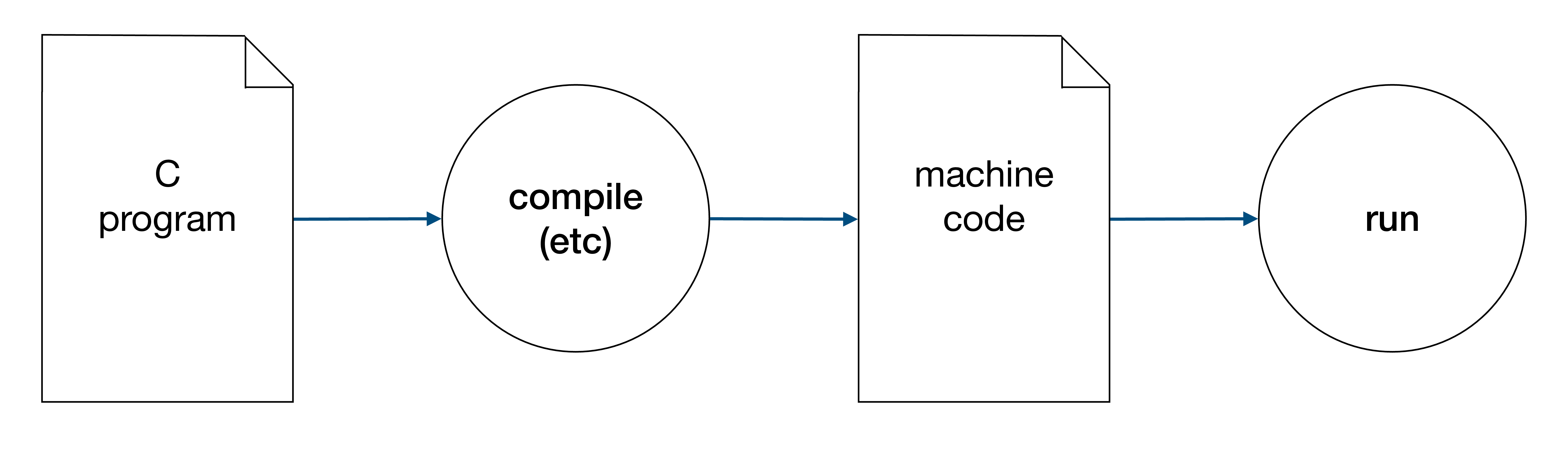] --- ### The `main` function - Entry point of a C program - Always return an `int` - Returns 0 if the program runs successfully. Non-zero otherwise. --- class: middle,center .w20[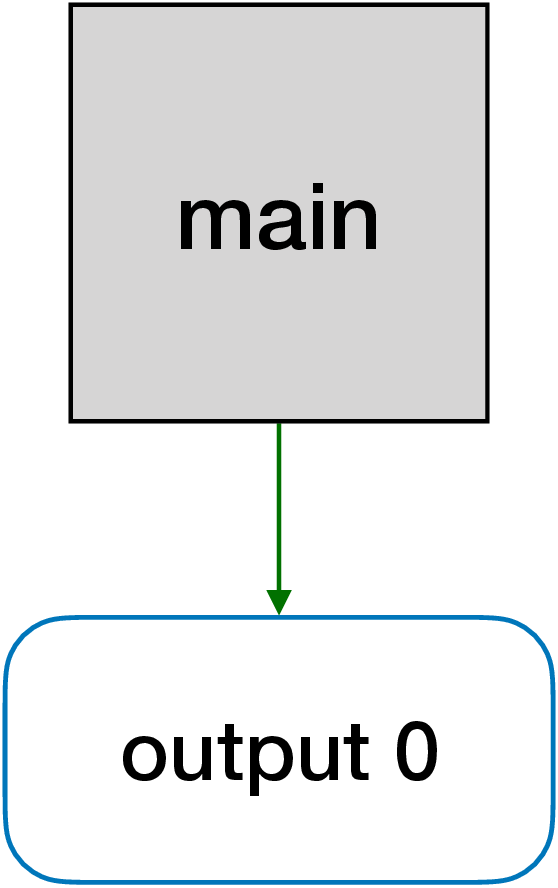] --- class: middle,center .fit[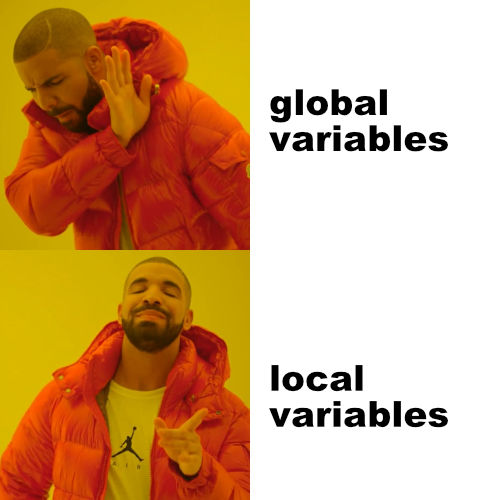] --- class: middle,center .tiny.center[ type | min bits | num of bits on PE nodes -----:|---------:|-----------------------: `char` | 8 | 8 `short` | 16 | 16 `int` | 16 | 32 `long` | 32 | 64 `long long` | 64 | 64 ] --- class: middle `int` is not sufficient for CS1010 purposes. We will only use `long` in CS1010. --- class: middle .tiny.center[ type | num of bits -----:|:-------- `float` | 32 `double` | 64 ] We will only use `double` in CS1010. --- class: middle,center .fit[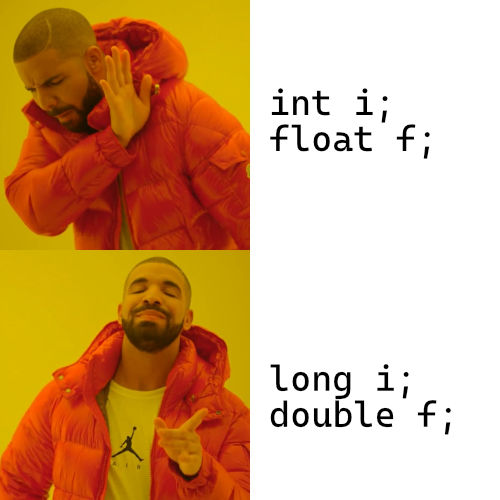] --- class: middle,center ### Basic operators `+` `-` `*` `/` `%` --- ### Pitfall: Order of operations can be confusing `8 / 2 * (2 + 2)` --- background-image: url("figures/ny-times-math-eq-stumps-internet.png") --- ### CS1010: Use parentheses everywhere `8 / (2 * (2 + 2))` `(8 / 2) * (2 + 2)` --- ### CS1010: No need to worry about
--- class: middle,center .fit[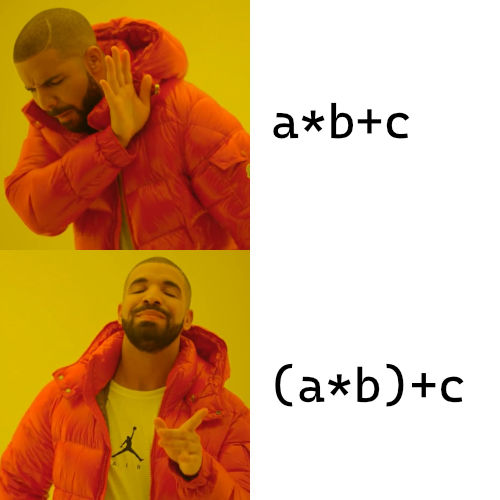] --- ### Pitfall: Integer Overflow ```C unsigned char c = 255; c = c + 1; ``` --- ### Pitfall: Integer Division ```C long three = 3; long two = 2; double one_half = three/two; ``` --- ### Pitfall: The % Operator `x % n` is equivalent to `x - ((x / n) * n)` - What is `-9 % 4`? - What is `9 % -4`? --- ### Compound Operator ```C x
= y; ``` is equivalent to ```C x = x
y; ``` E.g., `+=`, `-=`, `*=`, `/=`, `%=`, etc. --- ### Increment/Decrement Operator Postfix operator ```C x++; x--; ``` Use `x` first, then add/subtract one from `x`. --- ### Increment/Decrement Operator Prefix operator ```C ++x; --x; ``` Add/subtract one from `x`, use `x`. --- ### Increment/Decrement Operator ```C long x = 1; y = x++; // y becomes 1, x becomes 2 ``` --- ### Increment/Decrement Operator ```C long x = 1; y = ++x; // y becomes 2, x becomes 2 ``` --- ### Undefined Behavior ```C x = x++; x = x++ * ++x; ``` --- class: middle,center .fit[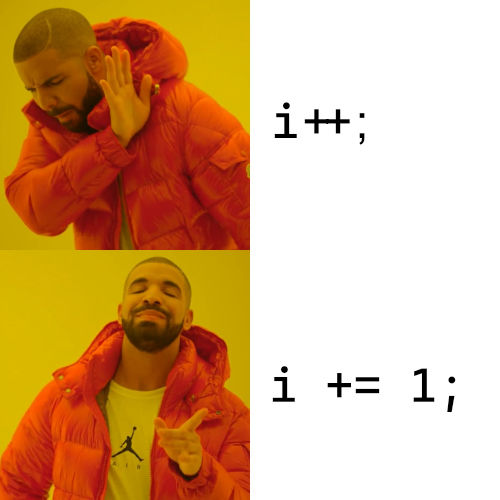] --- class: middle ### Programming cannot be learned by reading "Play" with code, ask "what if..", "why..", "why not..", etc --- ### Homework .small[ - Problem Set in [Unit 5](https://nus-cs1010.github.io/2425-s1/05-first-c.html#problem-set-5) - [Diagnostic Quiz for Lecture 3](https://canvas.nus.edu.sg/courses/62224/quizzes/40049) - [Exercise 0](https://nus-cs1010.github.io/2425-s1/exercises/ex00.html) ] --- class: bottom .tiny[ ]