class: middle, center # Lecture 5 ### 9 September 2024 **Admin Matters**
**Unit 11:** Loops
**Unit 12:** Loop Invariants
--- ### Programming Exercises - Exercise 1 due today - Exercise 2 released today - Past year PE questions released --- class: middle,center .fit[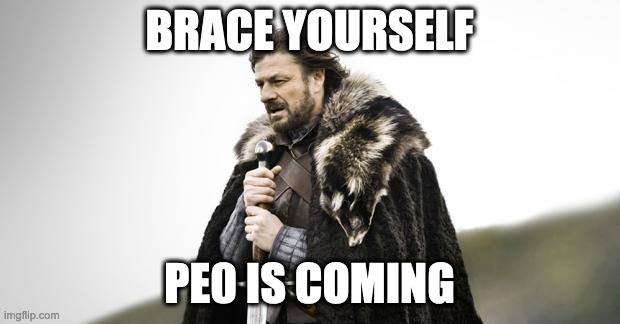] --- ### Practical Exam 0 - **Date**: 1 October 2024 (Tuesday) - **Time**: 6 - 9 PM - **Duration**: 2 hours - **Venue**: Programming labs in AS6/COM1/COM4 - **10%** of your grade - `ssh` into restricted PE hosts with special exam account using lab PCs --- - **Scope:** Units 1 - 12 / Exercises 0 - 2 - **Open Book** (printed/written notes only) - Past year questions on Canvas - File a ticket if you have a clash - More details in upcoming announcements --- class: center,middle ### Warning Pay attention to exam regulations. Disciplinary actions will be taken against violators. --- class: middle,center # Loops --- class: center,middle,wide .fit[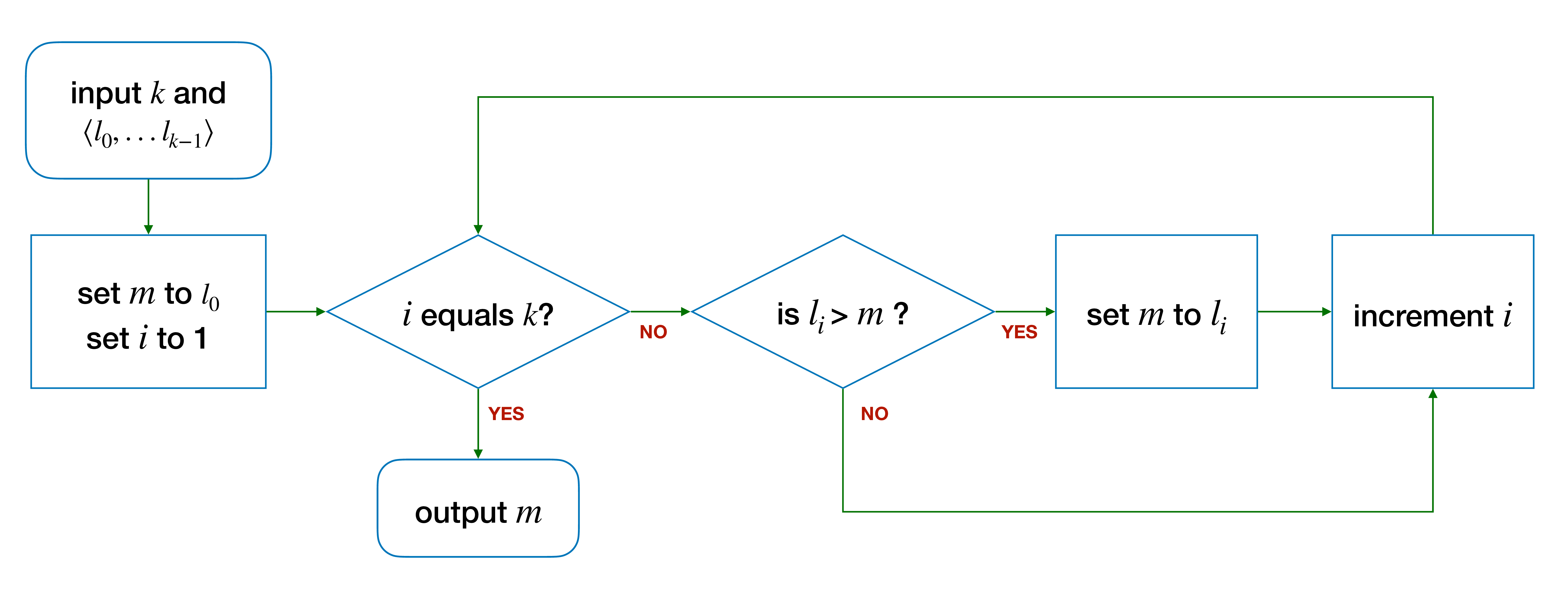] max(L,k) --- class: center,middle,wide .fit[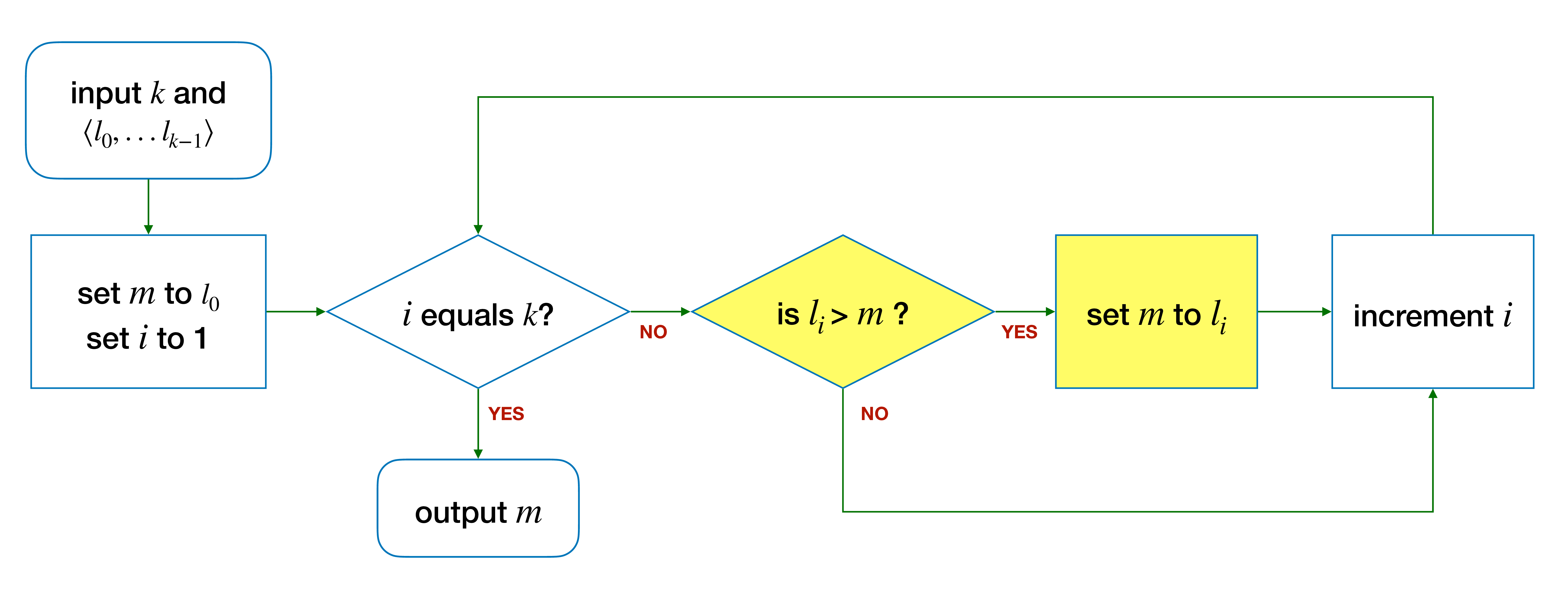] Loop body: what we do repeatedly --- class: center,middle,wide .fit[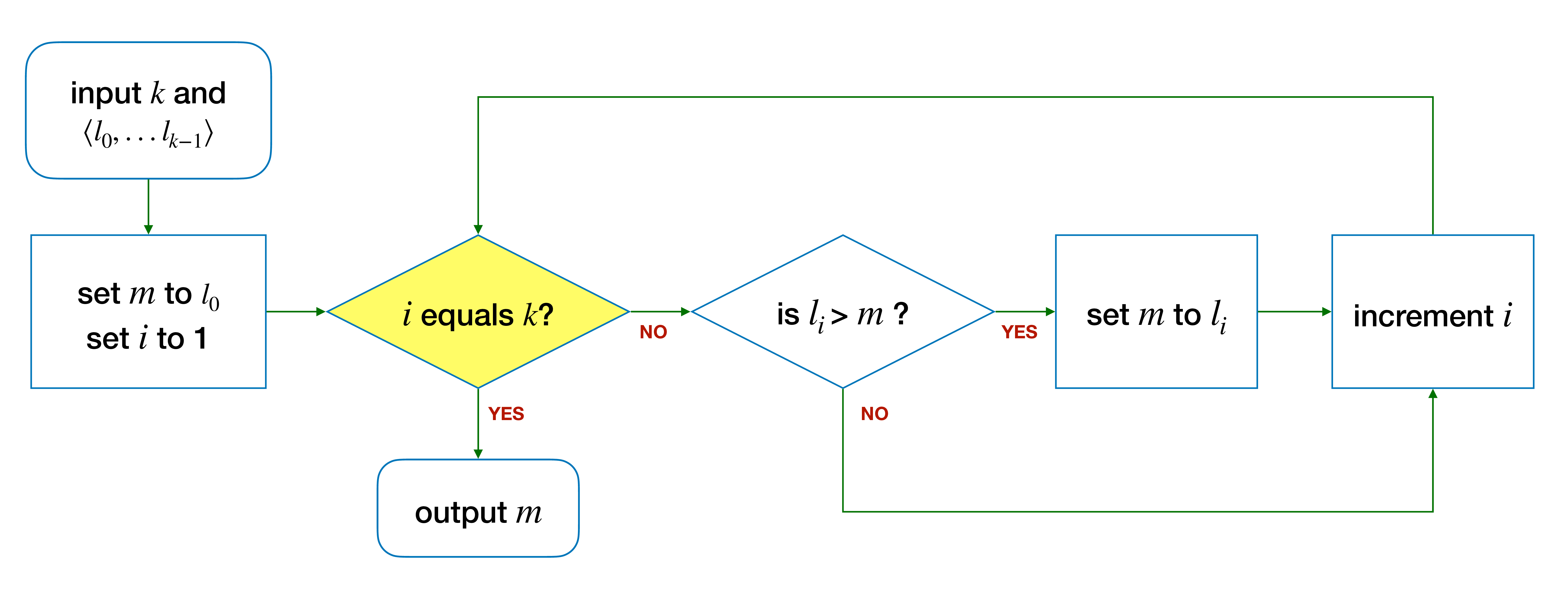] Terminating condition: when to stop repeating? --- class: center,middle,wide .fit[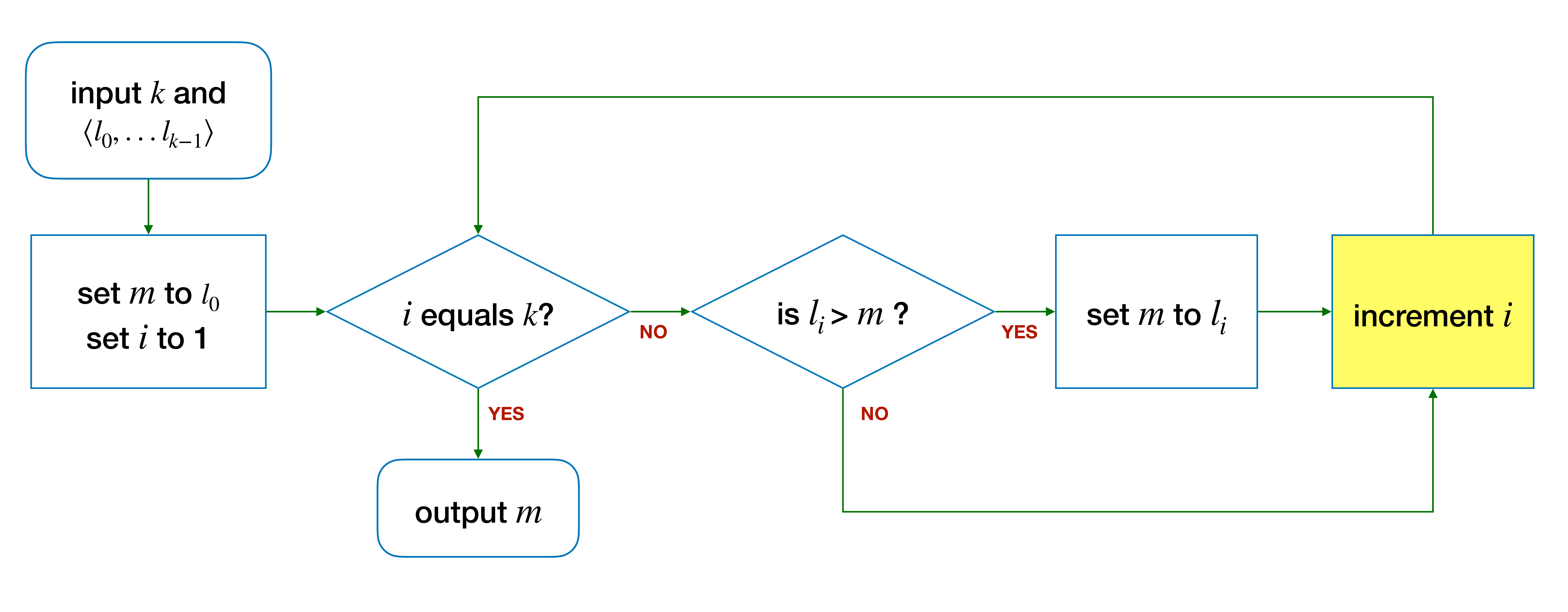] Loop update: what changes from one iteration to the next? --- class: center,middle,wide .fit[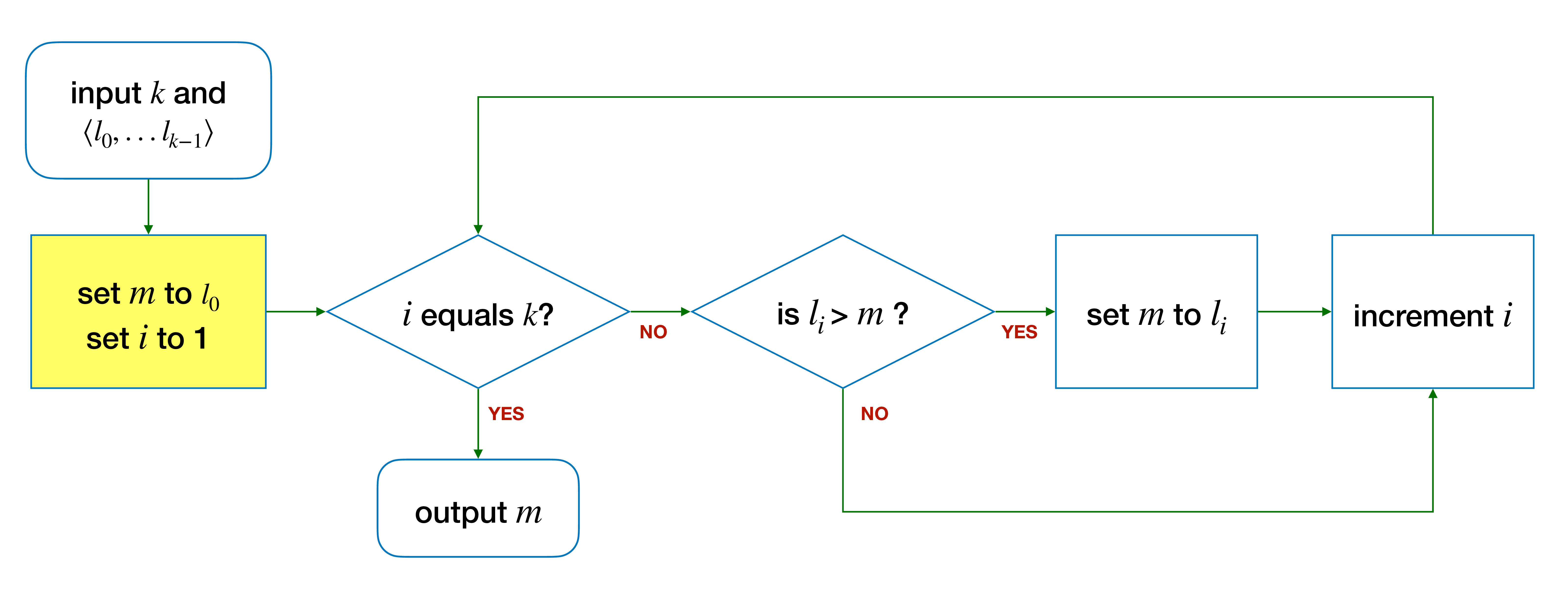] Loop initialization: what do we need to setup before entering the loop? --- class: center,middle,wide .fit[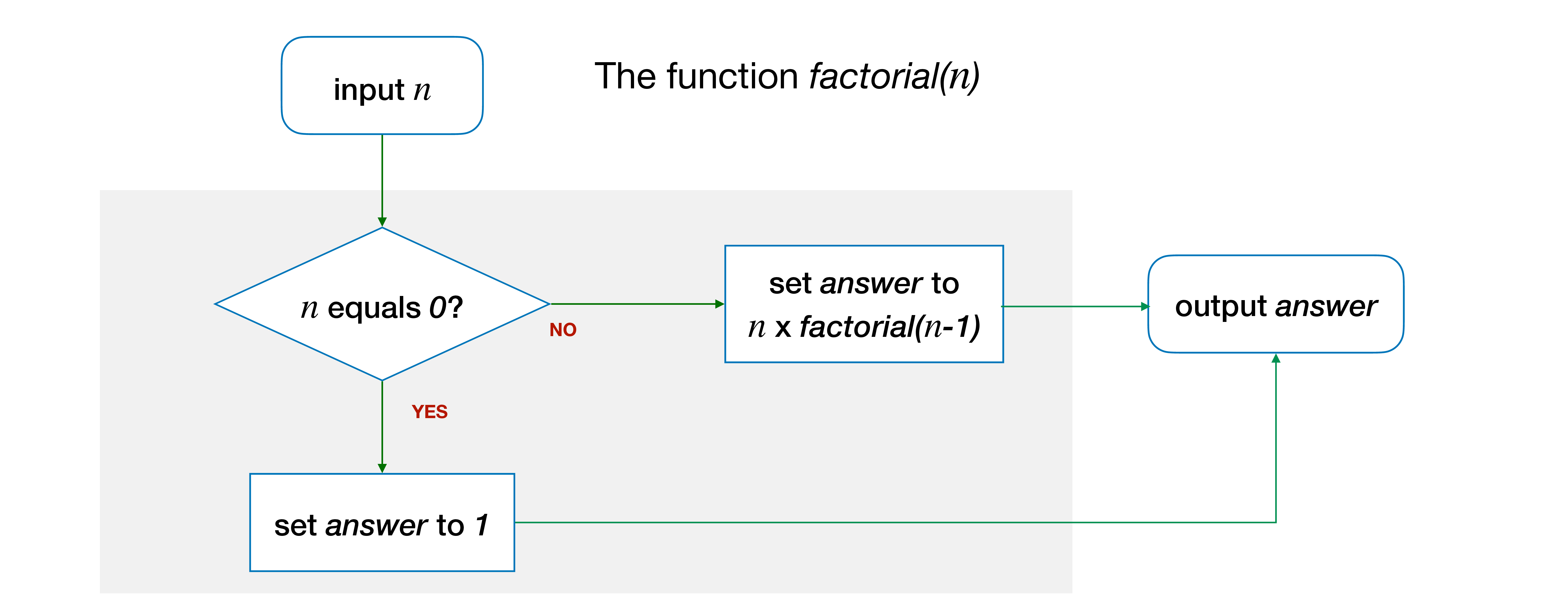] Finding factorial recursively --- --- class: center,middle,wide .fit[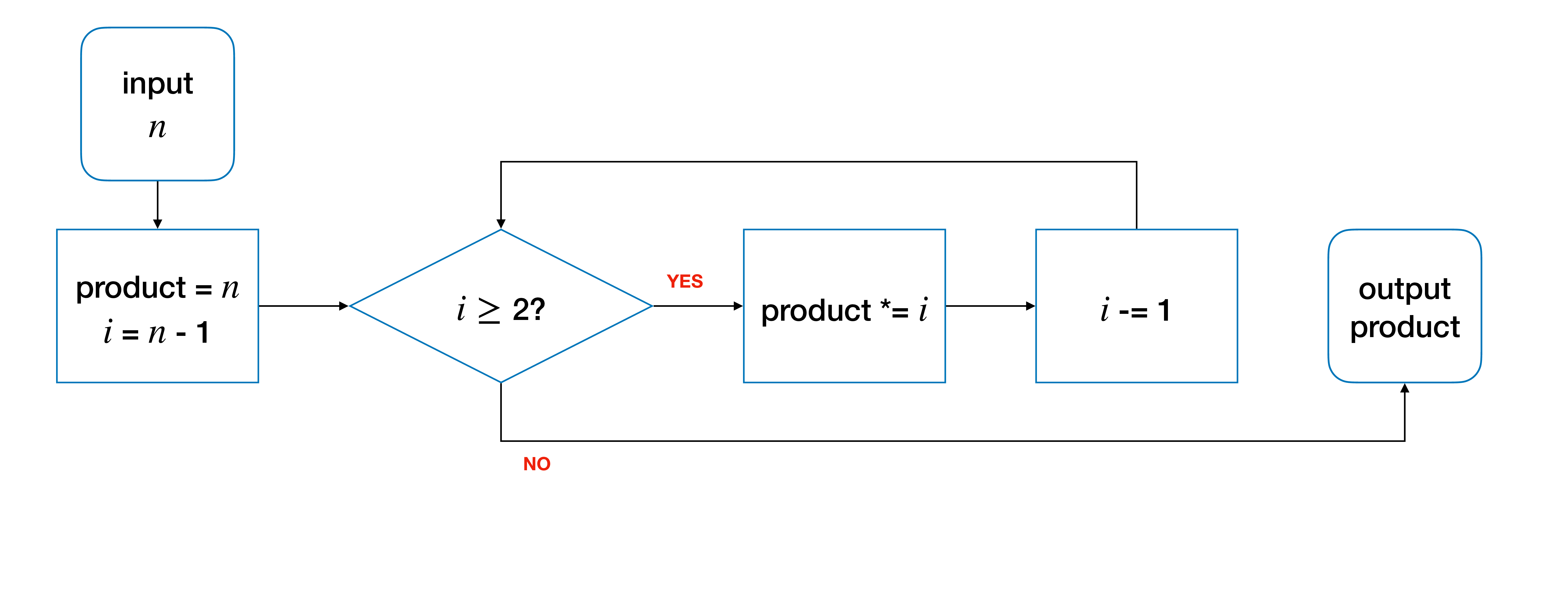] Finding factorial with a loop --- class: center,middle,wide .fit70[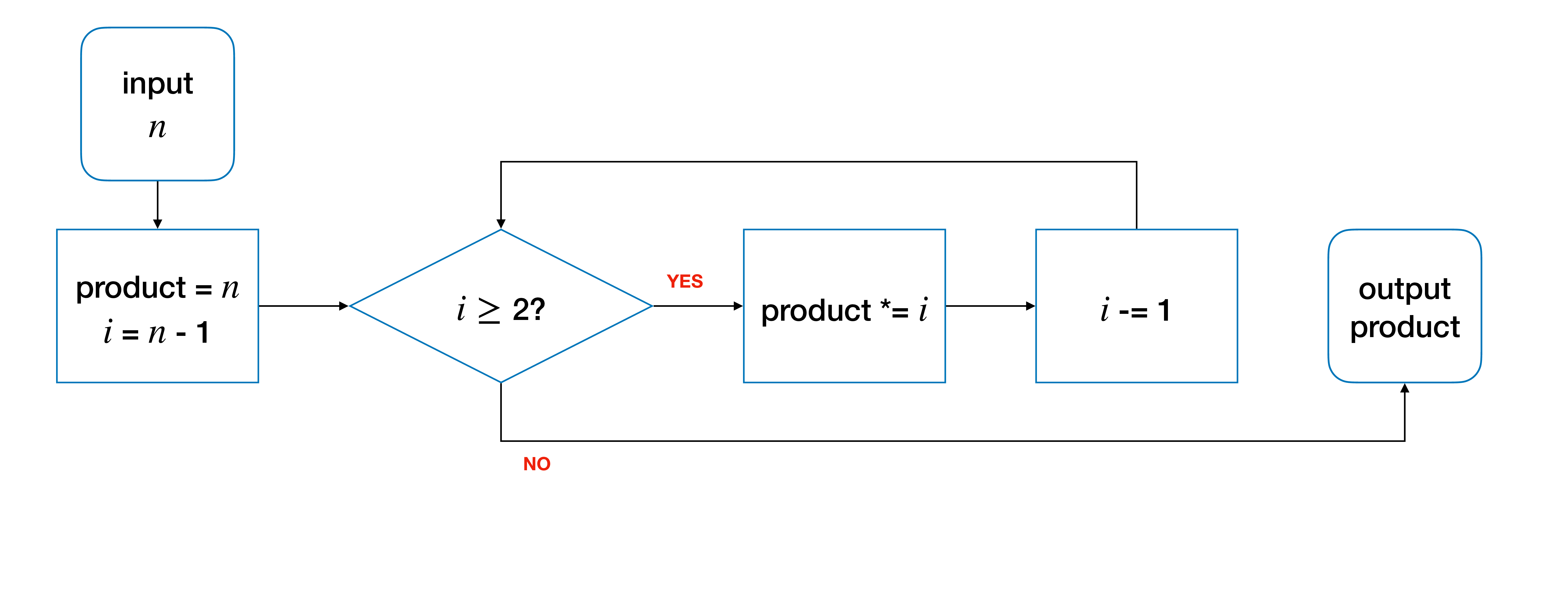] .fit70[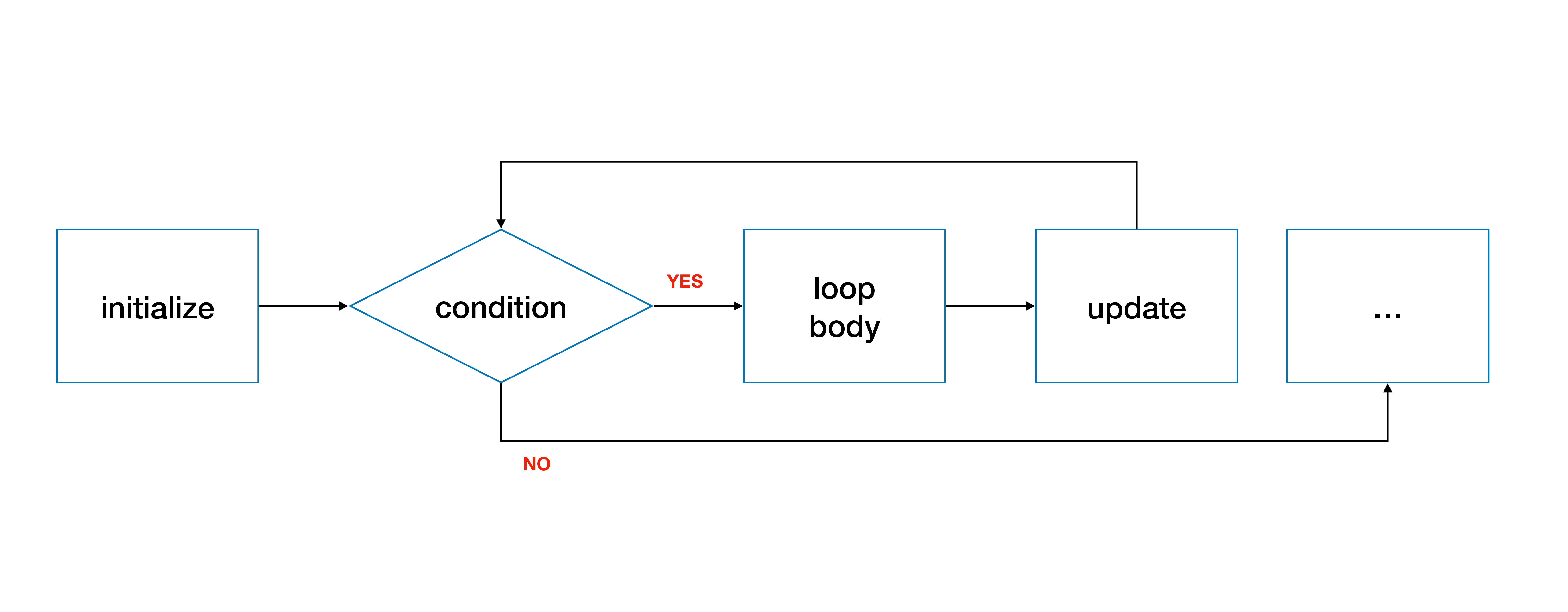] --- class: wide .fit80[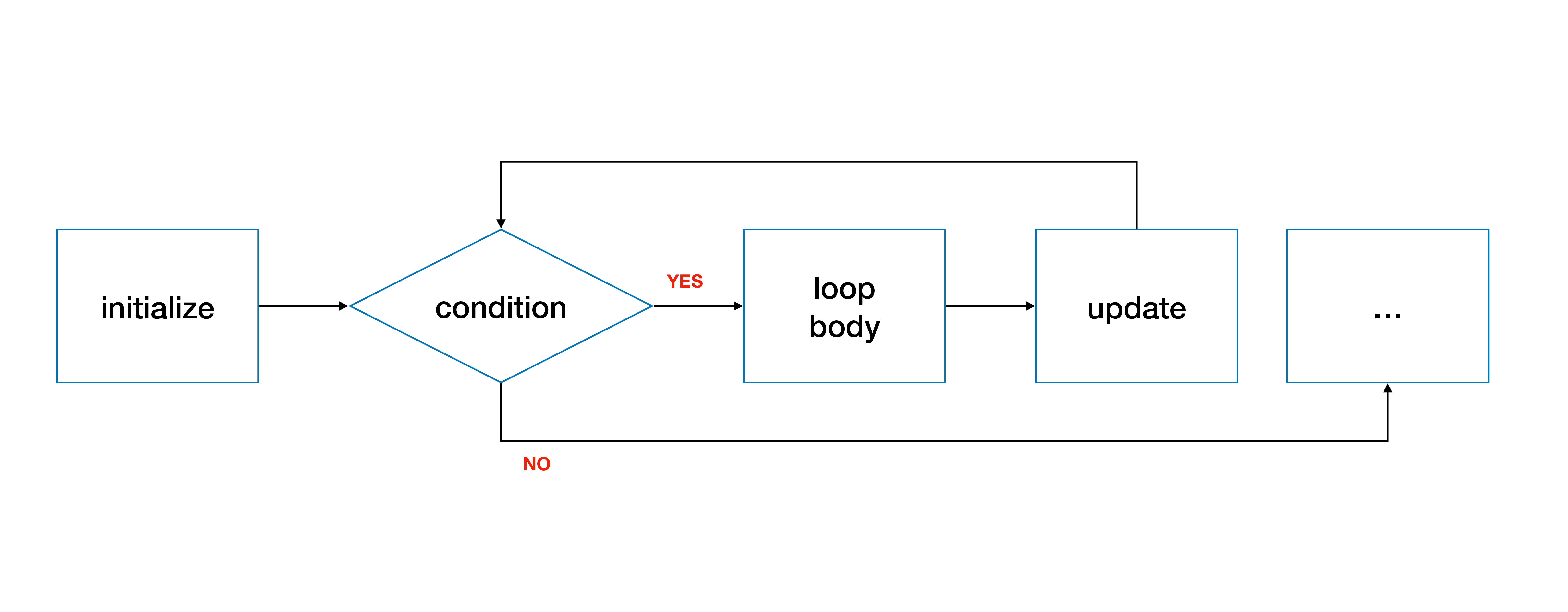] .small[ ```C for (initialize; condition; update) { body; } ``` ] --- class: wide .fit80[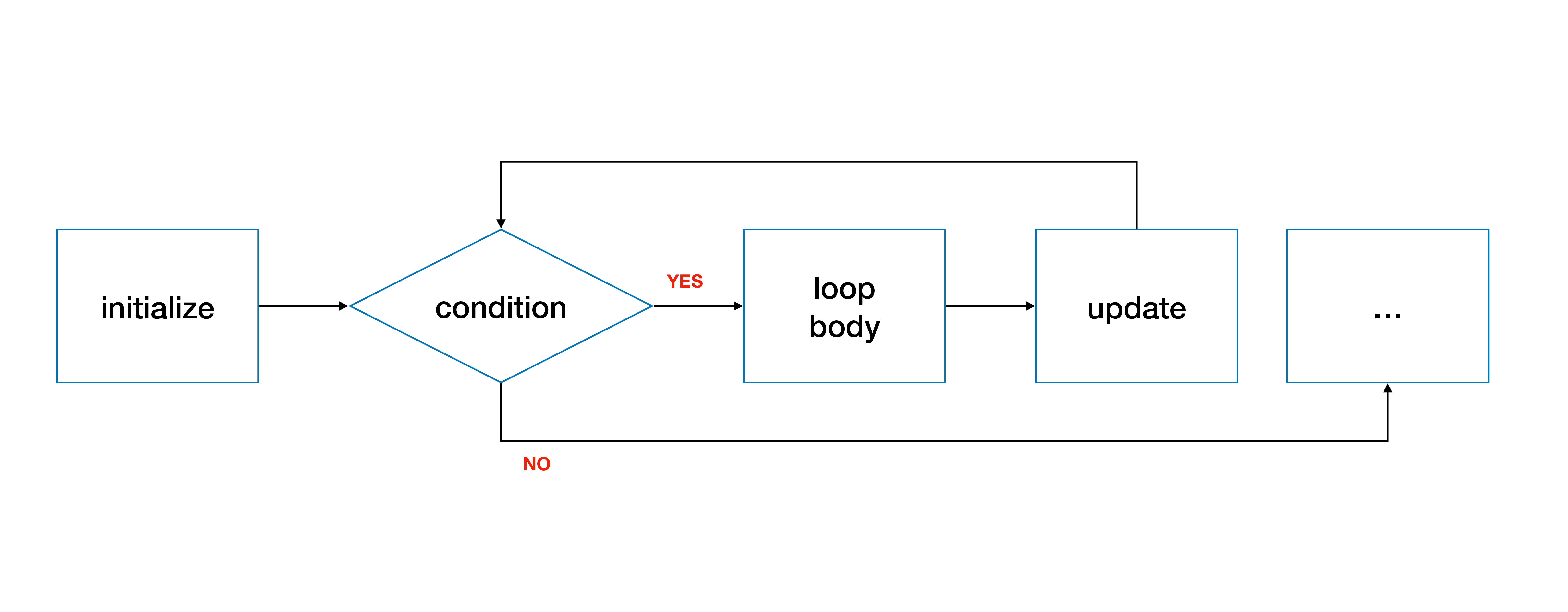] .small[ ```C initialize; while (condition) { body and update; } ``` ] --- class: center .fit[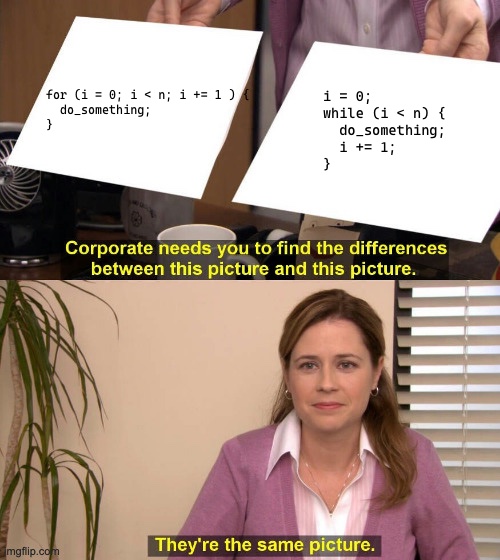] --- class: bottom,wide .fit80[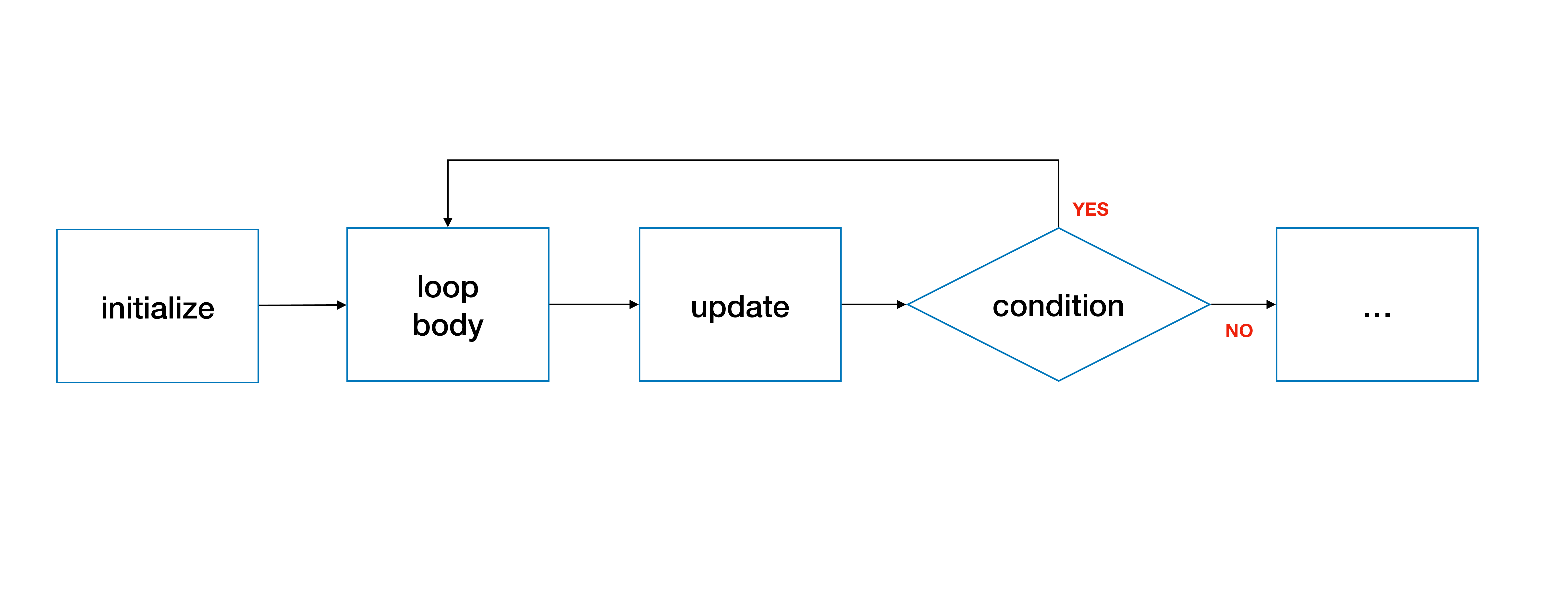] .small[ ```C initialize; do { body and update; } while (condition); ``` ] --- class: center, middle ### Demo: Number Guessing Game --- --- class: center,wide .fit[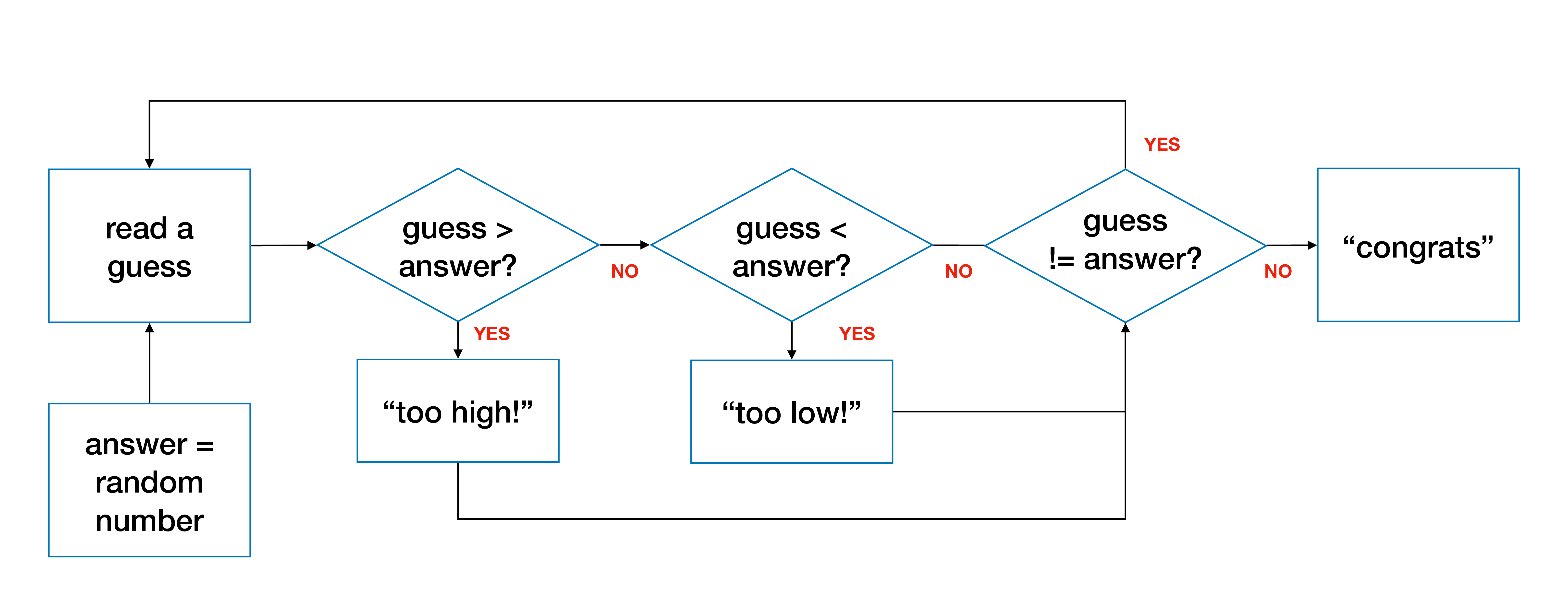] --- class: center, middle ## Infinite Loop --- ### For a Loop to Terminate Starting from an initial state, every update must move the state towards the termination state. --- class: middle,center ## Assertion ``` // { ... } ``` A logical expression that must be true at a given point in a program. --- Does this correctly compute factorial of $n$? .small[ ```C long factorial(long n) { long product = 1; long i = 1; while (i < n) { i += 1; product *= i; } return product; } ``` ] --- ```C while (cond) { // ?? : : } // ?? ``` --- ```C while (cond) { // { cond } <-- always true : : } // { !cond } <-- always true ``` --- ```C while (cond A) { // ?? : : // { cond B } } // ?? ``` --- ```C while (cond A) { // { A && B } : : // { cond B } } // { !A && B } ``` --- ```C // { cond B } while (..) { // ?? : : } // ?? ``` --- ```C // { cond B } while (..) { // B ❌ <-- only 1st loop : : } // B ❌ <-- only if loop is not entered ``` --- ```C // ?? while (cond A) { // ?? : : // ?? } // { cond B } <-- if we want this // where else must this be true? ``` --- ```C // { cond B } <-- must be true here while (cond A) { : : // { cond B } <-- and here } // { cond B } <-- to guarantee that // B is true here. ``` --- ### Loop Invariant An assertion that holds - before the loop; - at the end of each iteration of the loop; - after the loop; (but may be temporarily false during the loop) --- ### Loop Invariant .fit[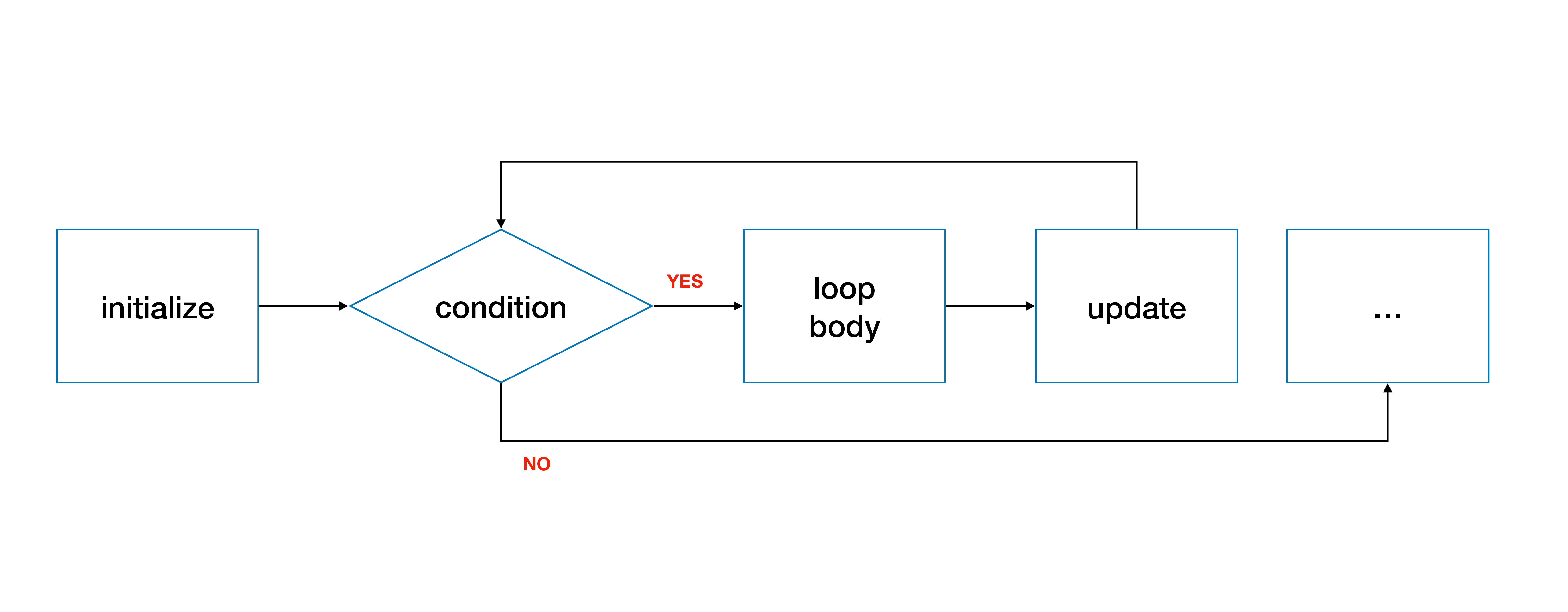] --- ### Loop Invariant An assertion that holds - before the loop; - at the end of 1st iteration - at the end of 2nd iteration - at the end of 3rd iteration : - after the loop; (but may be temporarily false during the loop) --- ### Loop Invariant An assertion that holds - before the loop; - at the end of 1st iteration - if holds at the end of $(k-1)$-th iteration, then also holds at the end of $k$-th iteration - after the loop; (but may be temporarily false during the loop) --- ### Loop Invariant An assertion that holds - before the loop; - at the end of 1st iteration - if holds at the beginning of $k$-th iteration, then also holds at the end of $k$-th iteration - after the loop; (but may be temporarily false during the loop) --- Show that `i >= 1` is a loop invariant. .small[ ```C long product = 1; long i = 1; // while (i < n) { // i += 1; product *= i; // } // ``` ] --- Show that `product >= 1` is a loop invariant. .small[ ```C long product = 1; long i = 1; // while (i < n) { // i += 1; product *= i; // } // ``` ] --- Show that `product == i!` is a loop invariant. .small[ ```C long product = 1; long i = 1; // while (i < n) { // i += 1; // product *= i; // } // ``` ] --- ### Homework - Self-Diagnostic Quiz for Lecture 5 - Exercise 2 - Problem Sets 11 and 12 --- class: bottom .tiny[ ]